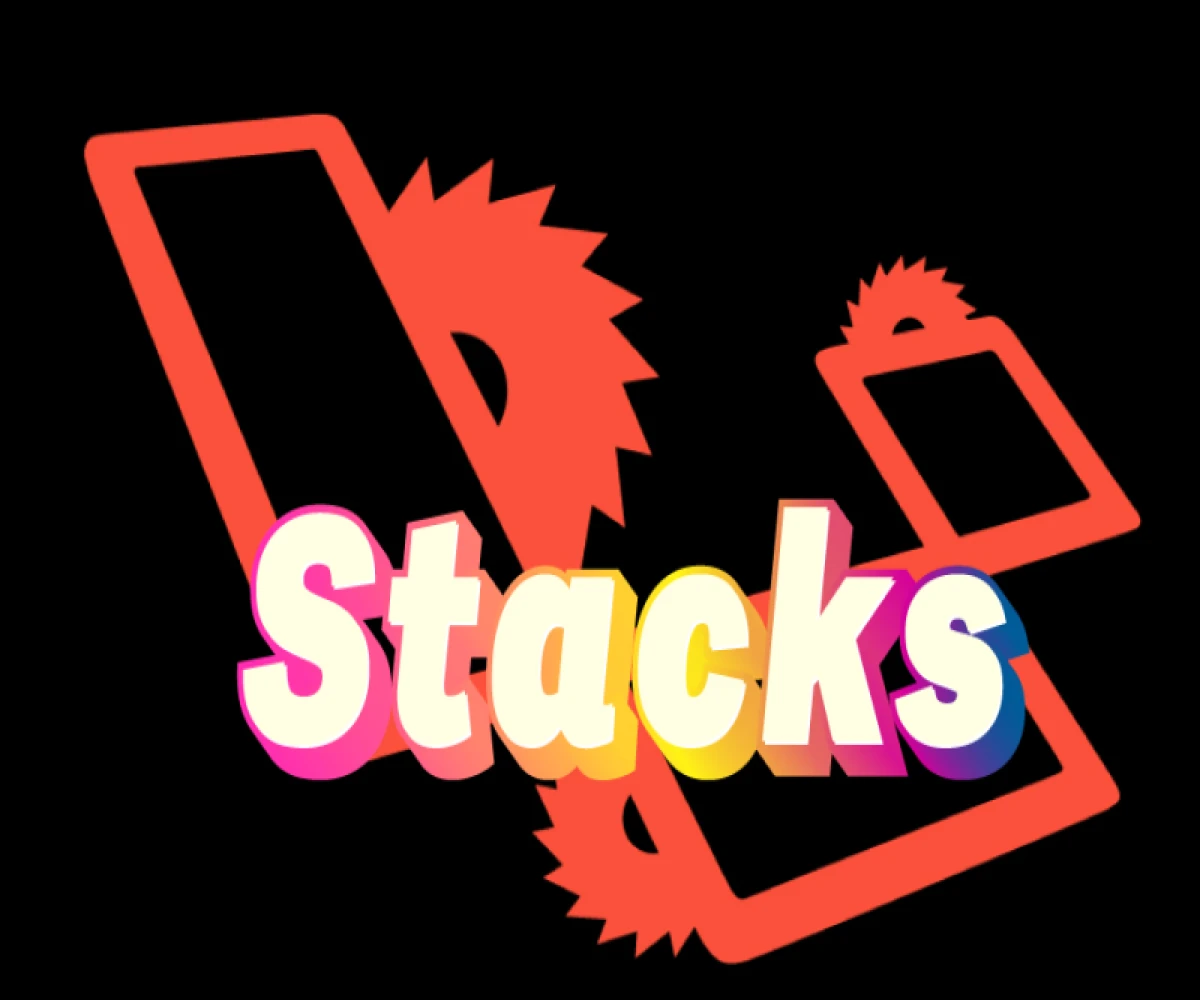
What is the stack directive in Laravel?
Understanding the Stack Directive in Laravel's Blade
Laravel's Blade templating engine offers a powerful feature called the stack directive. This directive helps manage content dynamically across various views, promoting code organization and reusability.
What is the Stack Directive?
The @stack
directive acts as a designated container within your Blade templates. It accumulates content pushed from other views using the corresponding @push
directive. This allows you to strategically insert content into specific sections of your layout without cluttering individual views.
The Power of Stacks
Stacks offer several advantages for your Laravel application:
- Improved Code Organization: By separating layout concerns from view-specific content, your code becomes cleaner and easier to maintain.
- Enhanced Reusability: You can define reusable content sections (like headers, footers, or sidebars) and inject them into various views using stacks.
- Flexible Content Management: Stacks provide a mechanism to dynamically add content from controllers or other parts of your application.
Using Stacks in Action
Here's a practical example demonstrating stacks:
1. Define Stacks in the Master Layout:
HTML
<head>
<title>My Website</title>
@stack('styles') </head>
<body>
@yield('content') @stack('scripts') </body>
2. Push Content from Child Views:
HTML
@push('styles')
<link rel="stylesheet" href="path/to/styles.css">
@endpush
@push('scripts')
<script src="path/to/scripts.js"></script>
@endpush
3. Render Stacks in the Master Layout:
The @stack
directive, placed strategically within your master layout, renders the accumulated content from the respective stacks defined earlier.
HTML
<head>
...
@stack('styles')
</head>
<body>
...
@stack('scripts')
</body>
In this example, the styles.css
and scripts.js
files are pushed from the child view and then included in the appropriate sections of the master layout using the @stack
directive.
Conclusion
The stack directive is a valuable tool in Laravel's Blade templating engine. By effectively utilizing stacks, you can create well-structured, maintainable, and reusable layouts for your web applications.
Additional Tips:
- Consider using
@prepend
to insert content at the beginning of a stack instead of appending. - You can define multiple stacks for different purposes (e.g., a stack for header scripts and another for footer scripts).
By incorporating stacks into your Laravel project, you'll experience a more organized and efficient development workflow.