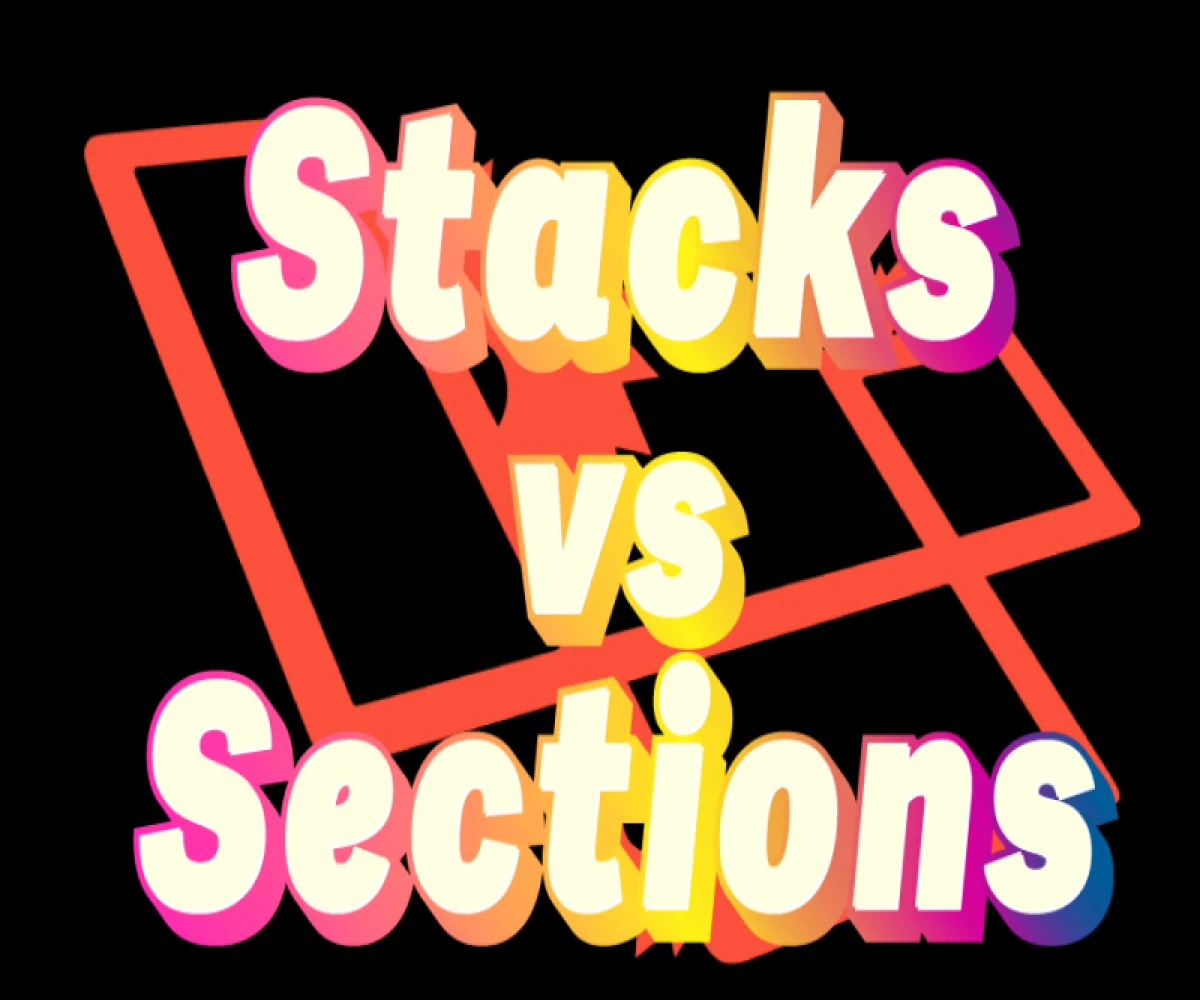
Sections vs. Stacks in Laravel Blade: The Battle of the Content Titans
Understanding Sections and Stacks in Laravel Blade
Laravel Blade, the powerful templating engine for Laravel applications, offers various tools for building clean and reusable layouts. Two key concepts for managing content within Blade templates are sections and stacks. While they share some similarities, they have distinct purposes:
Sections:
- Define named blocks of HTML content within a Blade template.
- Used for creating reusable content that can be injected into other templates.
- Defined with the
@section
directive and closed with@endsection
.
Example:
HTML
<header>
<h1>My Website</h1>
</header>
<main>
@yield('content')
</main>
<footer>
© {{ date('Y') }} My Company
</footer>
@extends('layouts.app')
@section('content')
<p>Welcome to the home page!</p>
@endsection
In this example, the app.blade.php
layout defines a content
section. The home.blade.php
content template extends the layout and fills the content
section with its specific content.
Stacks:
- Designed for accumulating content across multiple views.
- Useful for managing scripts, stylesheets, or other dynamic content.
- Content is added with the
@push
directive and displayed with the@stack
directive.
Example:
HTML
<head>
<title>@yield('title')</title>
@stack('styles')
</head>
<body>
@yield('content')
@stack('scripts')
</body>
@extends('layouts.app')
@section('title')
Home Page
@endsection
@push('styles')
<link rel="stylesheet" href="custom.css">
@endpush
@push('scripts')
<script src="home.js"></script>
@endpush
Here, the layout defines styles
and scripts
stacks. The home.blade.php
adds its specific CSS and JavaScript files to the respective stacks. This allows including scripts and styles unique to each view without cluttering the layout.
Key Differences:
- Inheritance: Sections are typically used within template inheritance, where child views fill sections defined in the parent layout. Stacks have no inheritance and can be used independently across views.
- Content Type: Sections are suitable for any HTML content, while stacks are primarily used for scripts, stylesheets, or other dynamic content intended for specific locations within the layout.
- Appending vs. Replacing: Content added to sections replaces any existing content within that section. With stacks, content is appended, allowing accumulation from various views.
Choosing Between Sections and Stacks:
- Use sections for reusable content that fits into designated areas of a layout.
- Use stacks for managing dynamic content like scripts and stylesheets that need to be included at specific points in the layout.
By understanding sections and stacks, you can create well-organized and flexible Laravel Blade templates, promoting code maintainability and reusability.