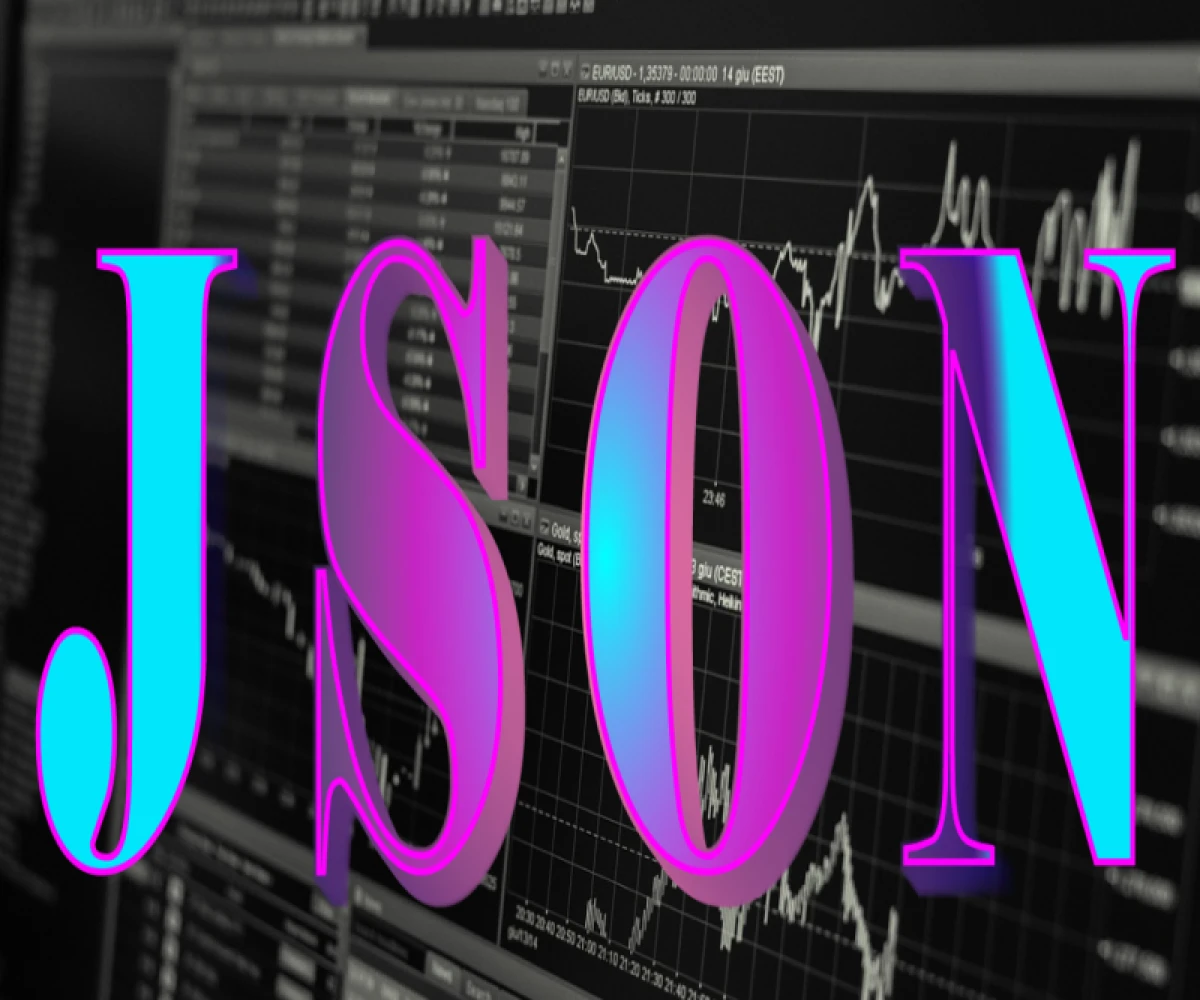
What is JSON?
Demystifying JSON: A Simple Guide to Data Exchange
In the realm of web development, exchanging information between different applications is crucial. This is where JSON (JavaScript Object Notation) steps in as a lightweight and human-readable format for structuring data.
What is JSON?
JSON stands for JavaScript Object Notation. Despite its name, JSON is a language-independent data format based on JavaScript object literals. It uses plain text to represent structured data in a way that's easy for both humans and machines to understand and process.
Why Use JSON?
Here's what makes JSON a popular choice for data interchange:
- Readability: JSON data is written in a clear and concise format, resembling JavaScript object syntax. This makes it easy for developers to comprehend and modify the data.
- Lightweight: Being plain text, JSON files are compact, leading to faster transmission times, especially when compared to bulkier formats like XML.
- Language Independence: Unlike formats tied to specific programming languages, JSON transcends language barriers. Various programming languages can parse and generate JSON data, making it a universal data exchange format.
- Simplicity: With only a few data types and a straightforward structure, JSON is relatively easy to learn and use.
Understanding JSON Structure
JSON data is built on two fundamental structures:
- Objects: An object represents a collection of key-value pairs. Keys are typically strings enclosed in double quotes, and values can be strings, numbers, booleans (true or false), null values, or even nested objects or arrays. Objects are denoted by curly braces
{}
.
For example:
JSON
{
"name": "Alice",
"age": 30,
"city": "New York"
}
In this example, "name", "age", and "city" are keys, and "Alice", 30, and "New York" are their corresponding values.
- Arrays: An array is an ordered list of values. These values can be of any JSON data type, including strings, numbers, booleans, null values, or even nested objects and arrays. Arrays are denoted by square brackets
[]
.
For example:
JSON
[
"apple",
"banana",
"orange"
]
Here, the array contains a list of three fruits: "apple", "banana", and "orange".
Nesting for Complex Data
JSON allows for nesting objects and arrays within each other, enabling the representation of complex data structures. Imagine a scenario where you want to represent customer information, including their name, address (which is itself an object with street and city), and a list of their previous purchases (which is an array of objects). Here's how you could achieve this using nested JSON:
JSON
{
"name": "Bob",
"address": {
"street": "123 Main St",
"city": "Seattle"
},
"purchases": [
{
"item": "Shirt",
"price": 25.99
},
{
"item": "Book",
"price": 14.50
}
]
}
Applications of JSON
JSON's versatility makes it a popular choice for various data exchange scenarios, including:
- APIs (Application Programming Interfaces): APIs often use JSON to transmit data between web applications.
- Client-Server Communication: JSON facilitates the exchange of data between web servers and client-side JavaScript code.
- Data Storage: JSON is a viable option for storing configuration settings or user data in a lightweight and manageable format.
Conclusion
JSON's simplicity, readability, and language independence have made it a cornerstone of modern web development. By understanding its structure and core concepts, you'll be well-equipped to leverage JSON for efficient data exchange in your web projects.
I hope this blog post clarifies JSON with examples. Feel free to explore further to delve deeper into its applications and practical usage within different programming languages.