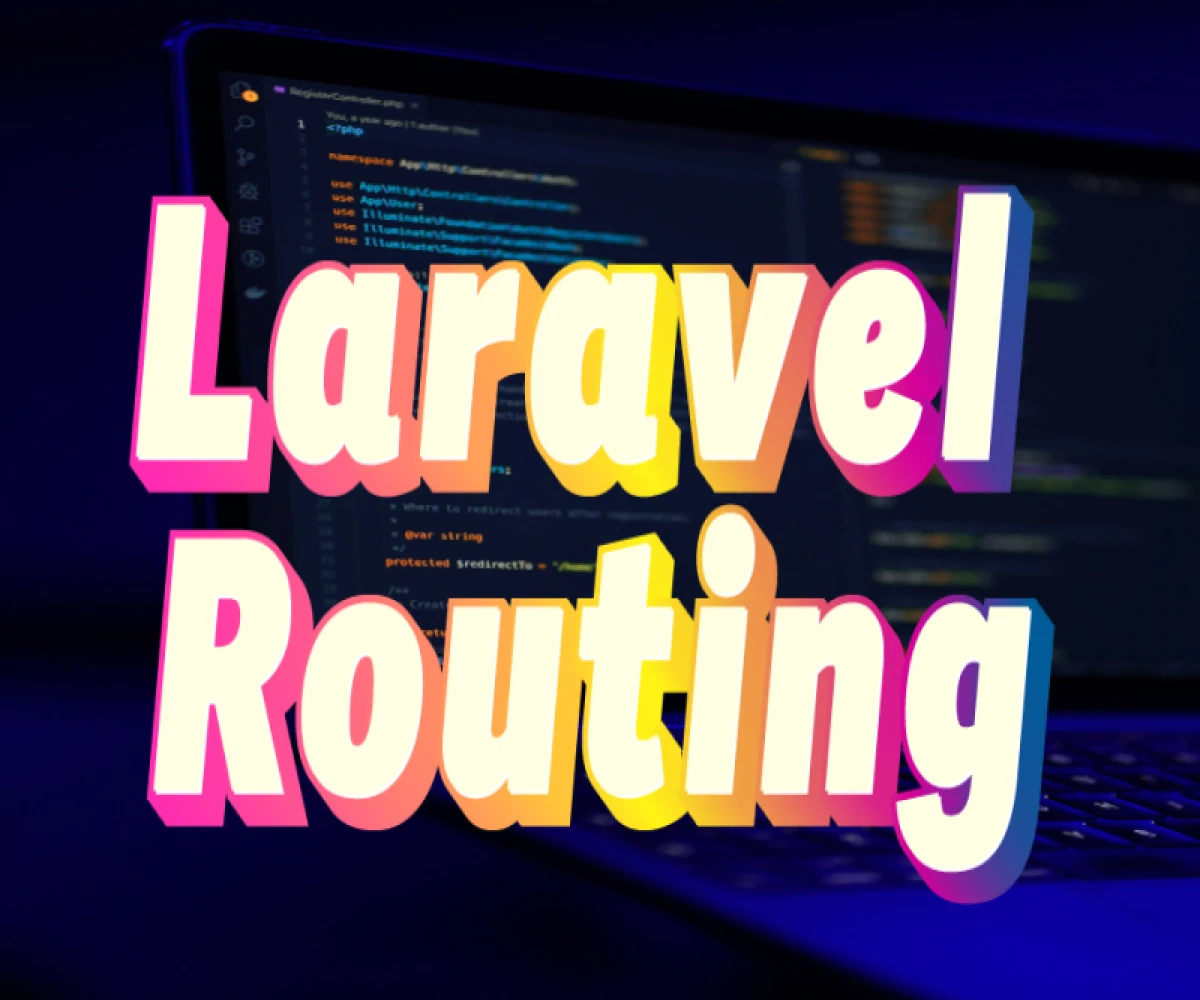
Build Organized Apps with Laravel Routing: Say Goodbye to Complexity
Laravel Routing: The Roadmap to Your Application
Laravel's routing system is the heart of your application, directing users to the appropriate functionality based on their requests. In this blog, we'll delve into the fundamentals of Laravel routing, understand the purpose of routes, explore different route types, and see how to define and handle requests in your Laravel project.
Understanding Routes: The Signposts of Your Application
Think of routes like signposts on a highway. They guide users (or in this case, their browsers) to specific destinations within your Laravel application. Each route is defined by a URL pattern and an action that handles the request. When a user visits a particular URL, Laravel matches it against defined routes to determine the appropriate action to execute.
Route Types: Catering to Different Actions
Laravel supports various route types, each corresponding to a specific HTTP method:
- GET: Used to retrieve data from the server. This is the most common type, typically used for displaying information on a webpage.
- POST: Used to submit data to the server, often for creating or updating information. Think of submitting a form.
- PUT: Used to update existing data on the server.
- PATCH: Similar to PUT, but used for partial updates.
- DELETE: Used to remove data from the server.
By defining different route types, you control how users interact with your application and manage data.
Defining Routes in Laravel: Taking Control
Laravel stores routes in PHP files located in the routes
directory. The primary file for web application routes is routes/web.php
. Here's the basic syntax for defining a route:
PHP
Route::method('/url', function () {
// Handle the request here
});
Route::method
: This specifies the HTTP method (GET, POST, etc.) the route handles./url
: This defines the URL pattern that matches the route.function()
: This is a closure (anonymous function) or a reference to a controller method that handles the request logic.
For example:
PHP
Route::get('/', function () {
return view('welcome'); // Returns the 'welcome' view
});
This route defines that when a user visits the root URL (/
), the welcome
blade template will be displayed.
Handling Incoming Requests: The Final Destination
The closure or controller method defined in the route handles the incoming request. It can perform various actions like retrieving data from a database, performing calculations, or generating a response. The response can be a simple string, a view template, or a JSON object, depending on the route's purpose.
By effectively utilizing Laravel's routing system, you can build a well-structured and organized application that efficiently handles user requests and delivers the desired functionality.