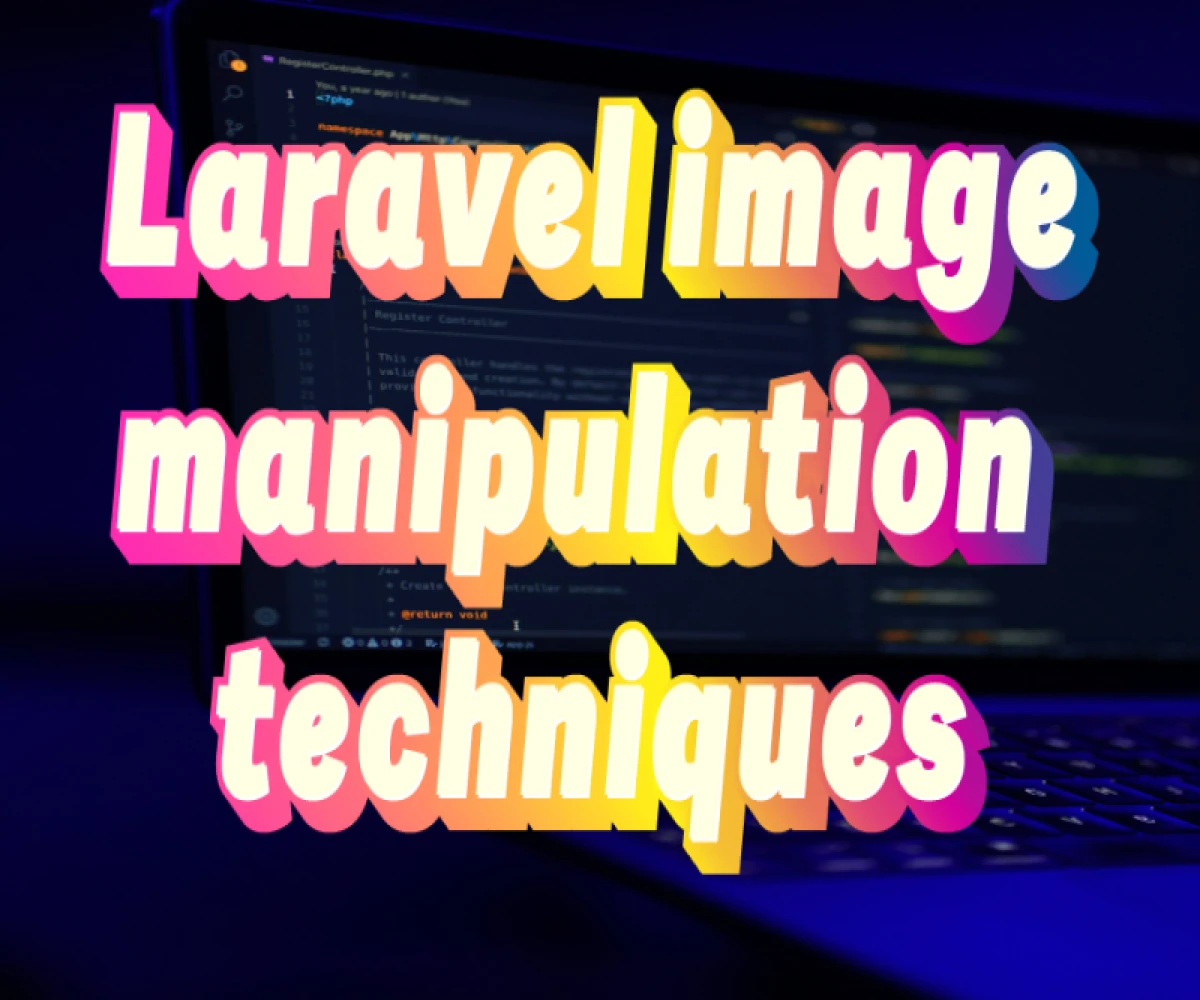
How to Fetch and Display Images in Laravel?
How to Fetch and Display Images in Laravel
Understanding the Basics
Before we dive into the code, let's clarify some fundamental concepts:
- Image Storage: Where will you store your images? Common options include:
- Public directory (accessible directly via web)
- Storage directory (requires configuration)
- Database (using a dedicated column, usually inefficient for large images)
- Image Retrieval: How will you access the image path from your model?
- Image Display: How will you render the image in your view?
Storing Images in the Public Directory
This is the simplest method for small-scale applications.
1. Create an Image Directory
Create a directory named public/images
in your Laravel project's root.
2. Upload Images
When uploading an image, save it to the public/images
directory. Here's a basic example:
PHP
public function store(Request $request)
{
$image = $request->file('image');
$imageName = time() . '.' . $image->getClientOriginalExtension();
$image->move(public_path('images'), $imageName);
// ... other logic
}
3. Display the Image
In your Blade view, use the asset
helper to generate the image path:
HTML
<img src="{{ asset('images/' . $product->image) }}" alt="Product Image">
Storing Images in the Storage Directory
For larger applications, storing images in the storage directory is recommended.
1. Configure Storage
In your .env
file, set the FILESYSTEM_DISK
to public
:
FILESYSTEM_DISK=public
2. Upload Images
Use the Storage
facade to store the image:
PHP
public function store(Request $request)
{
$image = $request->file('image');
$imageName = time() . '.' . $image->getClientOriginalExtension();
Storage::putFileAs('public/images', $image, $imageName);
// ... other logic
}
3. Display the Image
Use the Storage
facade to generate the image URL:
HTML
<img src="{{ Storage::url('public/images/' . $product->image) }}" alt="Product Image">
Using Laravel Media Library (Recommended)
For complex image management, consider using the Spatie Media Library package. It offers advanced features like multiple conversions, different storage drivers, and more.
1. Installation
Bash
composer require spatie/laravel-medialibrary
2. Model Setup
PHP
use Spatie\MediaLibrary\HasMedia;
use Spatie\MediaLibrary\InteractsWithMedia;
class Product extends Model implements HasMedia
{
use InteractsWithMedia;
public function registerMediaCollections(): void
{
$this->addMediaCollection('images');
}
}
3. Uploading Image
PHP
$product->addMedia($image)->toMediaCollection('images');
4. Displaying Image
HTML
<img src="{{ $product->getFirstMediaUrl('images') }}" alt="Product Image">
Additional Considerations
- Image Optimization: Use image optimization techniques to reduce file size.
- Image Formats: Choose appropriate image formats (JPEG, PNG, SVG) based on image content.
- Image Alt Text: Provide descriptive alt text for accessibility.
- Image Responsiveness: Use responsive image techniques for different screen sizes.
By following these guidelines, you can effectively fetch and display images in your Laravel applications.