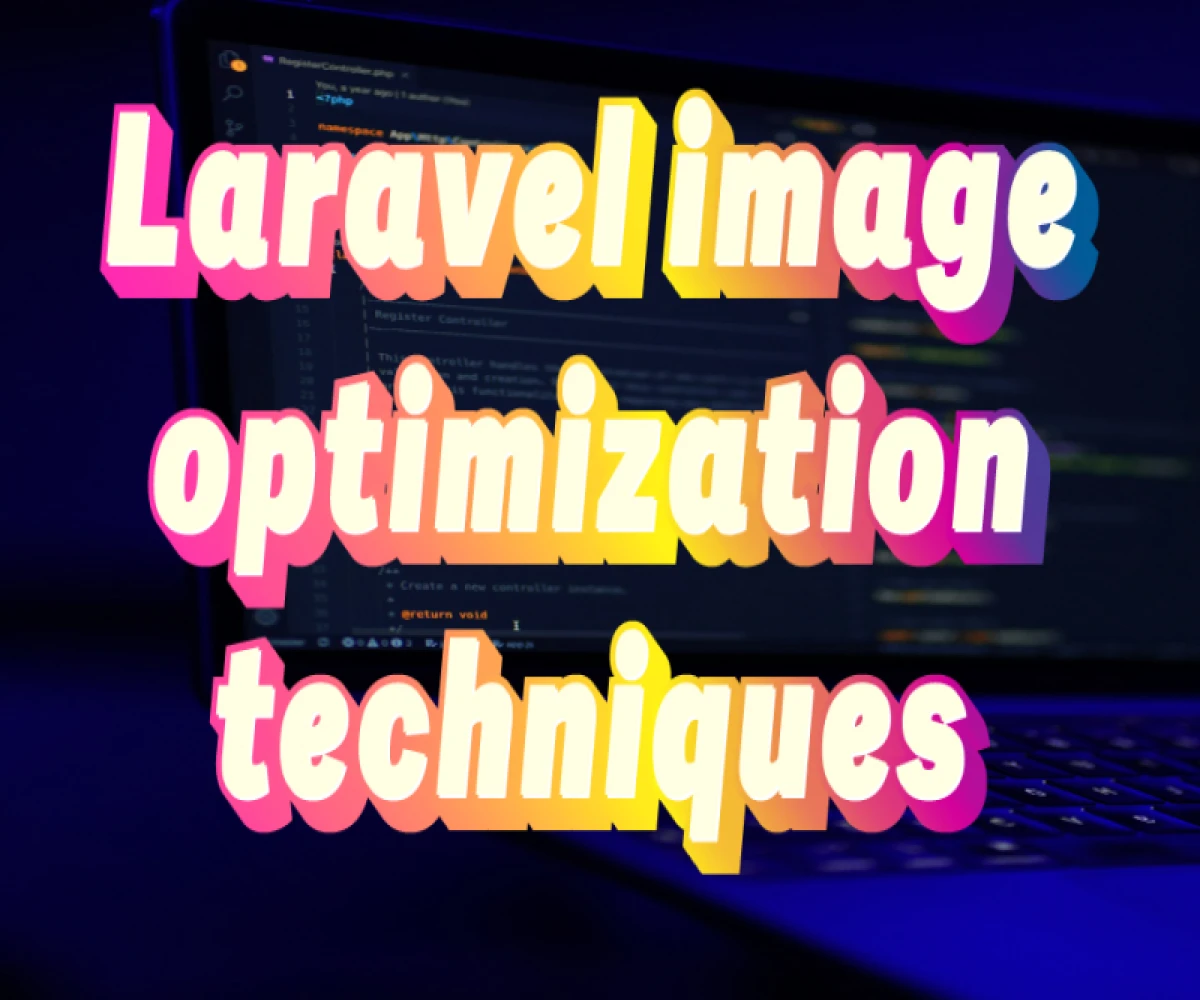
How to Reduce Image Quality in Laravel ?
Laravel
2 months ago
How to Reduce Image Quality in Laravel
Understanding the Need
Reducing image quality is crucial for optimizing website performance and reducing loading times. Large image files can significantly impact user experience. Laravel provides effective tools to handle image manipulation, allowing you to compress images without compromising visual appeal.
Using Intervention Image
Intervention Image is a popular Laravel package for image manipulation. It offers various methods to resize, crop, and adjust image quality.
Installation
Bash
composer require intervention/image
Basic Usage
PHP
use Intervention\Image\ImageManagerStatic as Image;
// Load an image
$image = Image::make('path/to/image.jpg');
// Reduce quality to 70%
$image->quality(70);
// Save the image
$image->save('path/to/compressed_image.jpg');
Complete Example
PHP
use Illuminate\Http\Request;
use Intervention\Image\ImageManagerStatic as Image;
public function store(Request $request)
{
$image = $request->file('image');
$imageName = time() . '.' . $image->extension();
// Resize and compress the image
$img = Image::make($image->path())
->resize(800, 600, function ($constraint) {
$constraint->aspectRatio();
})
->quality(70)
->save(public_path('images/' . $imageName));
// Save the image path to the database
// ...
}
Additional Considerations
- Quality vs. File Size: Experiment with different quality levels to find the optimal balance between image quality and file size.
- Image Format: Consider using JPEG for photographs and PNG for images with transparency.
- Progressive JPEG: This format displays a low-resolution version of the image first, improving perceived loading speed.
- Image Optimization: Tools like TinyPNG or Squoosh can further compress images.
- Lazy Loading: Load images only when they are about to be displayed.
- Image CDN: Use a Content Delivery Network to distribute image files across multiple servers, improving load times.
Advanced Techniques
- Image Optimization Packages: Explore packages like
spatie/image
for more advanced features and optimizations. - Custom Image Processing: For complex image manipulations, consider writing custom image processing logic using GD or Imagick.
- Server-Side Rendering (SSR): Pre-render images on the server to reduce client-side processing.
Conclusion
By effectively reducing image quality and implementing optimization techniques, you can significantly enhance your Laravel application's performance and user experience. Remember to strike a balance between image quality and file size to meet your specific requirements.