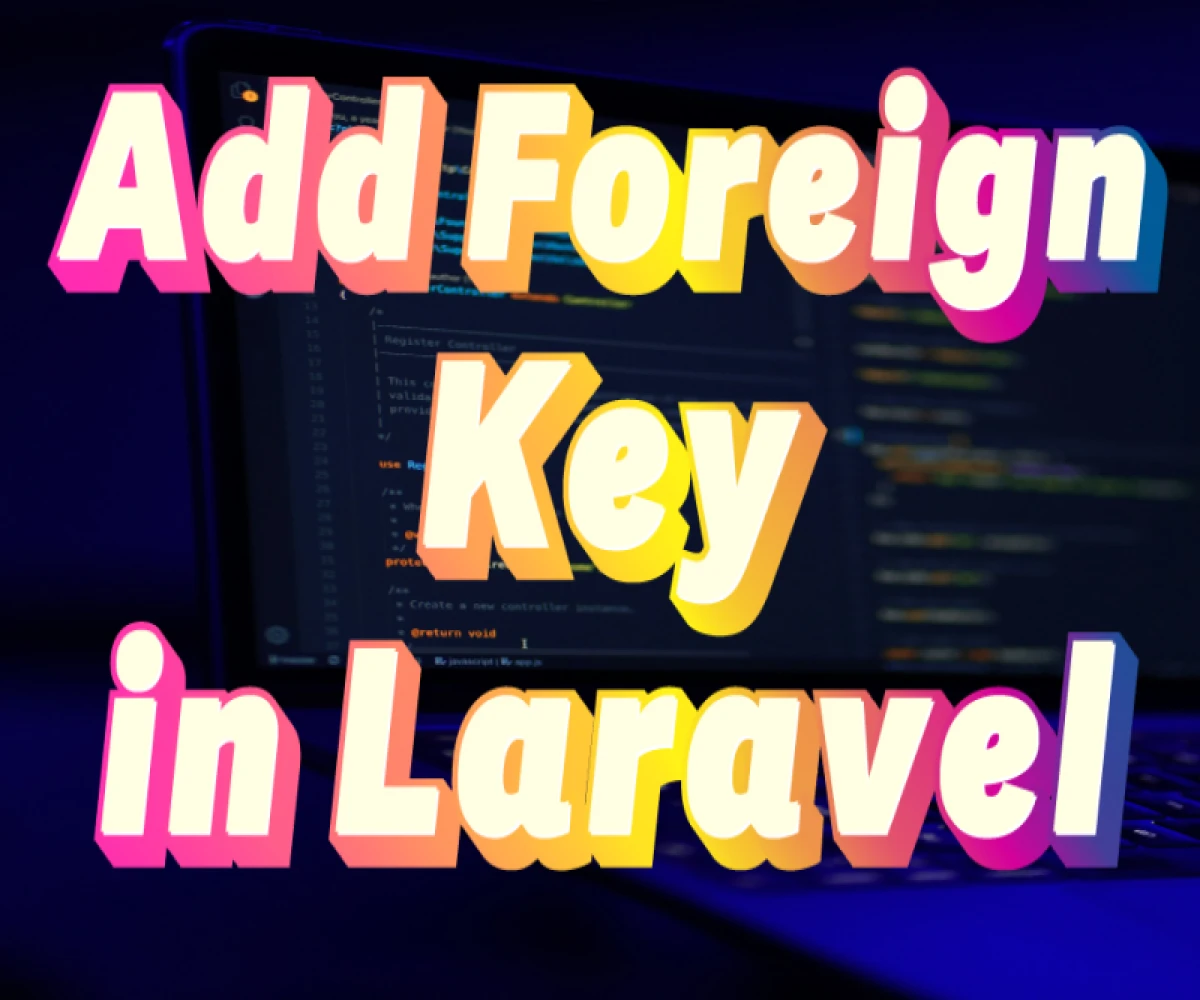
How to add a foreign key to an existing table in Laravel?
Laravel
4 months ago
In Laravel applications, foreign keys play a crucial role in ensuring data integrity and modeling the relationships between your database tables. This tutorial guides you through adding a foreign key to an existing table in Laravel, exploring different methods.
Methods:
-
Migration: (Recommended for most cases)
- Create a new migration file using
php artisan make:migration AddAuthorForeignKeyToPosts
. - Inside the
up
method, use theSchema::table
facade:
PHP
Schema::table('posts', function (Blueprint $table) { // Add the foreign key column (ensure it's the correct data type) $table->unsignedInteger('author_id')->nullable(); // Define the foreign key constraint $table->foreign('author_id') ->references('id') // Replace 'id' with the actual primary key of the referenced table ->on('users') // Replace with the referenced table name ->onDelete('cascade'); // Optional: Set deletion behavior (cascade, set null, etc.) });
- Run migrations using
php artisan migrate
.
- Create a new migration file using
-
Raw SQL: (Use with caution, especially in production)
- Execute a raw SQL statement using Laravel's DB facade:
PHP
DB::statement("ALTER TABLE posts ADD CONSTRAINT fk_posts_author_id FOREIGN KEY (author_id) REFERENCES users(id) ON DELETE CASCADE;");
-
Schema Builder: (For more complex modifications)
- Create a new migration and use the
Schema::table
facade:
PHP
Schema::table('posts', function (Blueprint $table) { $table->unsignedBigInteger('author_id')->nullable()->after('content'); // Position the new column // Define the foreign key constraint $table->foreign('author_id') ->references('id') ->on('users') ->onDelete('cascade'); });
- Create a new migration and use the
Important Considerations:
- Existing Data: If your
posts
table already has data, ensure the newauthor_id
column allows null values initially. You might need to update existing data to reference valid user IDs in theusers
table. - Data Type Compatibility: The data type of the
author_id
column (unsignedInteger
in this example) must match the data type of the primary key (id
) in theusers
table. - Testing: Thoroughly test your application after adding the foreign key constraint to ensure the relationship between posts and authors is enforced correctly.
By following these steps and understanding the considerations, you can effectively add foreign keys to your existing tables in Laravel!