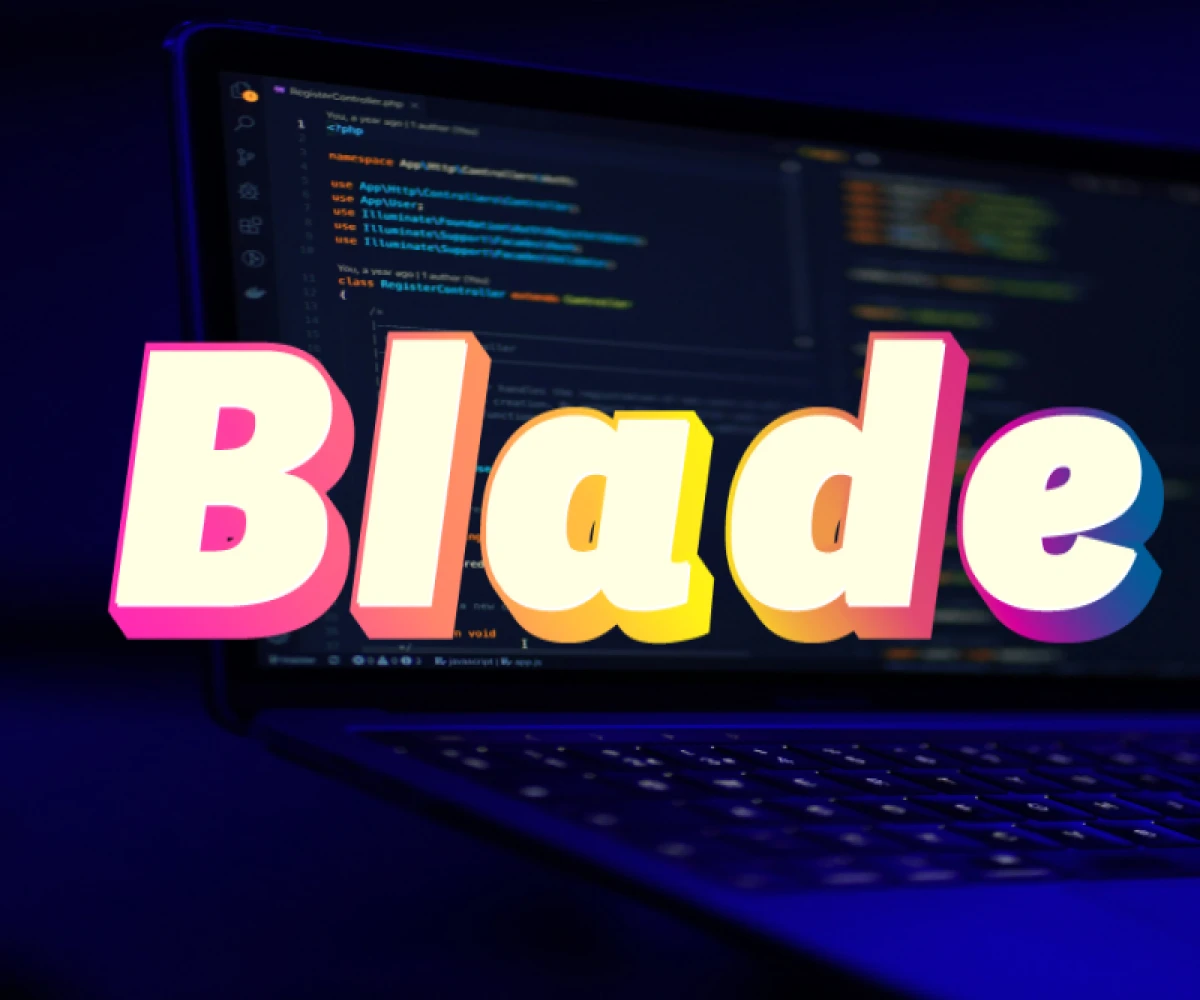
Goodbye Messy Code: Mastering Laravel Views with Blade
Crafting Dynamic Views with Laravel's Blade Templating Engine
Laravel, a popular PHP framework, streamlines web development with its built-in templating engine: Blade. Blade offers a powerful yet straightforward approach to creating dynamic HTML views, separating presentation logic from application code. This blog post dives into Blade's core concepts and demonstrates its basic syntax for crafting flexible web pages.
Embracing Dynamic Views with Blade
Imagine a website displaying a list of products. Each product requires unique information like name, description, and price. Traditional PHP would involve embedding this data directly within HTML, leading to messy and unmaintainable code. Blade solves this by separating the HTML structure (the view) from the data retrieval logic (the controller). This separation promotes cleaner code and easier updates.
Here's how Blade achieves dynamic views:
- Blade Templates: Create files with the
.blade.php
extension typically stored in theresources/views
directory. These files contain HTML markup sprinkled with Blade directives. - Data Passing: Laravel controllers pass data (variables, arrays, objects) to the Blade views. This data becomes accessible within the templates.
- Rendering Views: The Blade template engine processes the directives and data, generating the final HTML sent to the browser.
Mastering Basic Blade Syntax
Blade directives are instructions that begin with the @
symbol. Let's explore some fundamental directives:
- @if and @else: Control flow directives used for conditional statements.
HTML
@if ($user->isAdmin)
<p>Welcome Admin!</p>
@else
<p>Hello, {{ $user->name }}!</p>
@endif
This example checks if the $user
has an isAdmin
property set to true
. If so, it displays an admin message. Otherwise, it displays a personalized greeting using the $user->name
variable.
- @foreach: Iterates through collections of data.
HTML
@foreach ($products as $product)
<div class="product">
<h2>{{ $product->name }}</h2>
<p>{{ $product->price }}</p>
</div>
@endforeach
This loop iterates over a $products
collection, displaying each product's name and price within a product container.
- @include: Includes another Blade template within the current one, promoting reusability.
HTML
<header>
@include('layouts.header')
</header>
<main>
</main>
<footer>
@include('layouts.footer')
</footer>
Here, the @include
directive incorporates the header.blade.php
and footer.blade.php
templates, promoting a consistent header and footer across multiple pages.
These are just a taste of Blade's capabilities. With Blade, you can craft dynamic and reusable views, keeping your Laravel applications clean and maintainable. Explore the official Laravel documentation for a comprehensive list of directives and features to elevate your web development experience with Blade.