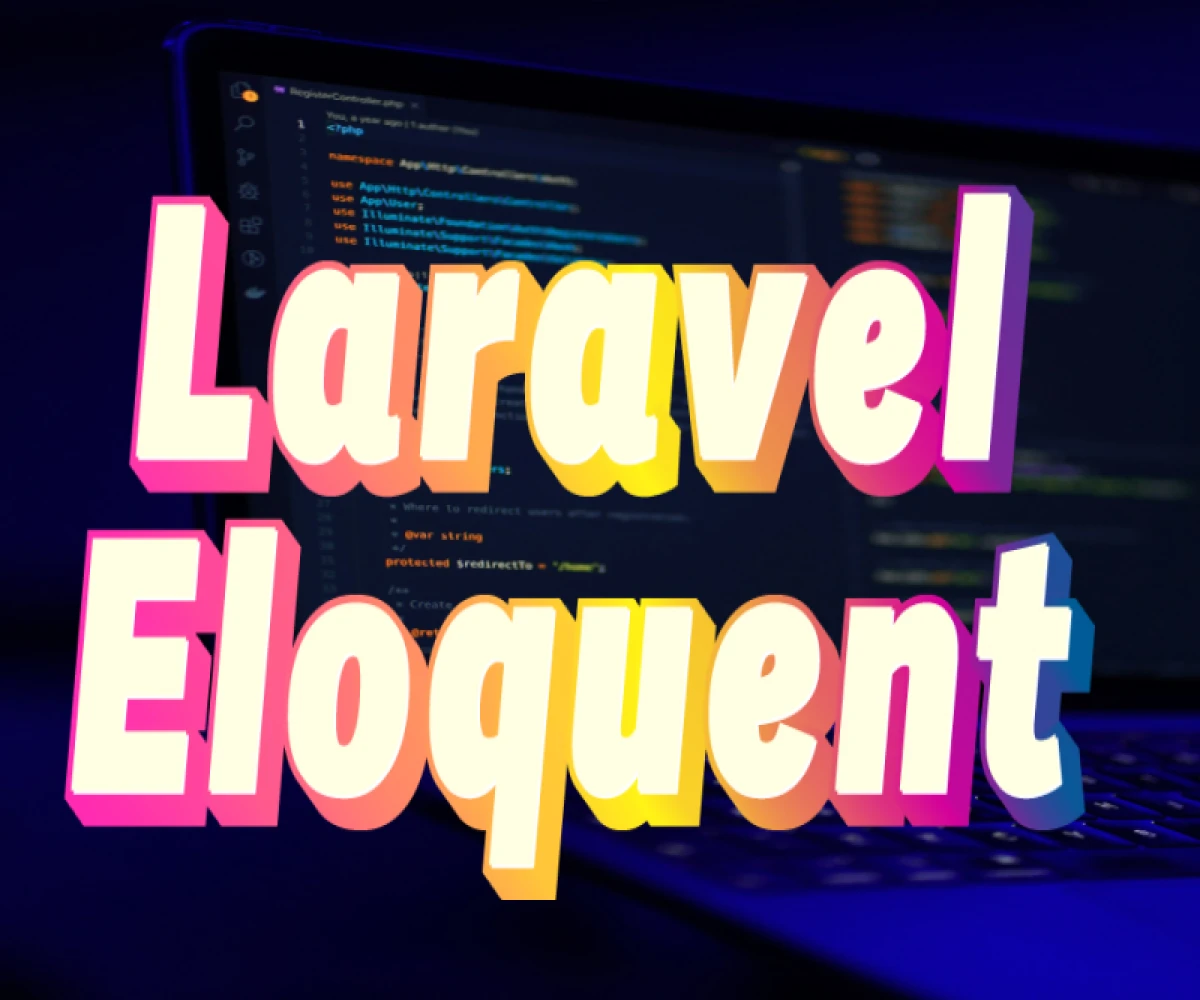
Don't Write Raw SQL Again: Laravel Eloquent to the Rescue!
Laravel Eloquent ORM: A Deep Dive
Laravel Eloquent is a powerful Object-Relational Mapper (ORM) that simplifies interacting with your database in Laravel applications. It provides an intuitive way to work with database tables through PHP objects, reducing the need for writing raw SQL queries.
This tutorial will take you on a deep dive into Eloquent, covering:
- Models: Defining models that represent your database tables.
- Relationships: Understanding and implementing one-to-one, one-to-many, and many-to-many relationships between models.
- Eloquent Methods: Exploring essential methods for CRUD (Create, Read, Update, Delete) operations and other database interactions.
1. Models: The Bridge Between PHP and Database
Eloquent models are PHP classes that represent your database tables. Each model maps to a single table, and its properties correspond to the table's columns. Here's how to create a model:
PHP
<?php
namespace App\Models;
use Illuminate\Database\Eloquent\Model;
class User extends Model
{
// Define fillable attributes for mass assignment protection
protected $fillable = ['name', 'email', 'password'];
}
In this example, the User
model represents the users
table. The $fillable
property defines the attributes that can be mass assigned during data insertion or update.
2. Relationships: Connecting Your Data
Eloquent allows you to define relationships between models, reflecting the connections between your tables. Here are the common types:
- One-to-One: A single record in one table relates to a single record in another. Example: A
User
has oneProfile
.
PHP
class User extends Model
{
public function profile()
{
return $this->hasOne(Profile::class);
}
}
- One-to-Many: A single record in one table relates to many records in another. Example: A
User
has manyPosts
.
PHP
class User extends Model
{
public function posts()
{
return $this->hasMany(Post::class);
}
}
- Many-to-Many: Many records in one table relate to many records in another. Example: A
Post
has manyTags
, and aTag
has manyPosts
. This requires a pivot table to manage the relationships.
PHP
class Post extends Model
{
public function tags()
{
return $this->belongsToMany(Tag::class);
}
}
These are just basic examples. Eloquent provides methods for defining inverse relationships, defining custom pivot tables, and working with polymorphic relationships.
3. Eloquent Methods for Database Interaction
Eloquent offers a wide range of methods for interacting with your database:
- CRUD Operations:
create()
- Creates a new record in the database.find($id)
- Retrieves a record by its primary key.first()
- Retrieves the first record matching a condition.get()
- Retrieves all records from a table.update()
- Updates an existing record.delete()
- Deletes a record.
- Querying:
where()
- Filters results based on conditions.orderBy()
- Orders results by a specific column.limit()
andskip()
- Limit and skip results for pagination.
- Relationships:
with()
- Eager loading related models with a single query.
- Other Methods:
save()
- Saves a model to the database (insert or update).firstOrFail()
- Retrieves the first record or throws an exception if not found.
By chaining these methods, you can build complex queries and interact with your database effectively.
Additional Resources:
- Laravel Eloquent Documentation: https://laravel.com/docs/11.x/eloquent
- A Practical Introduction to Laravel Eloquent ORM: https://www.youtube.com/watch?v=EPUpOhgTdAU
This tutorial provides a foundation for understanding Laravel Eloquent. As you delve deeper, explore the extensive documentation and resources available to master this powerful tool for building database-driven Laravel applications.