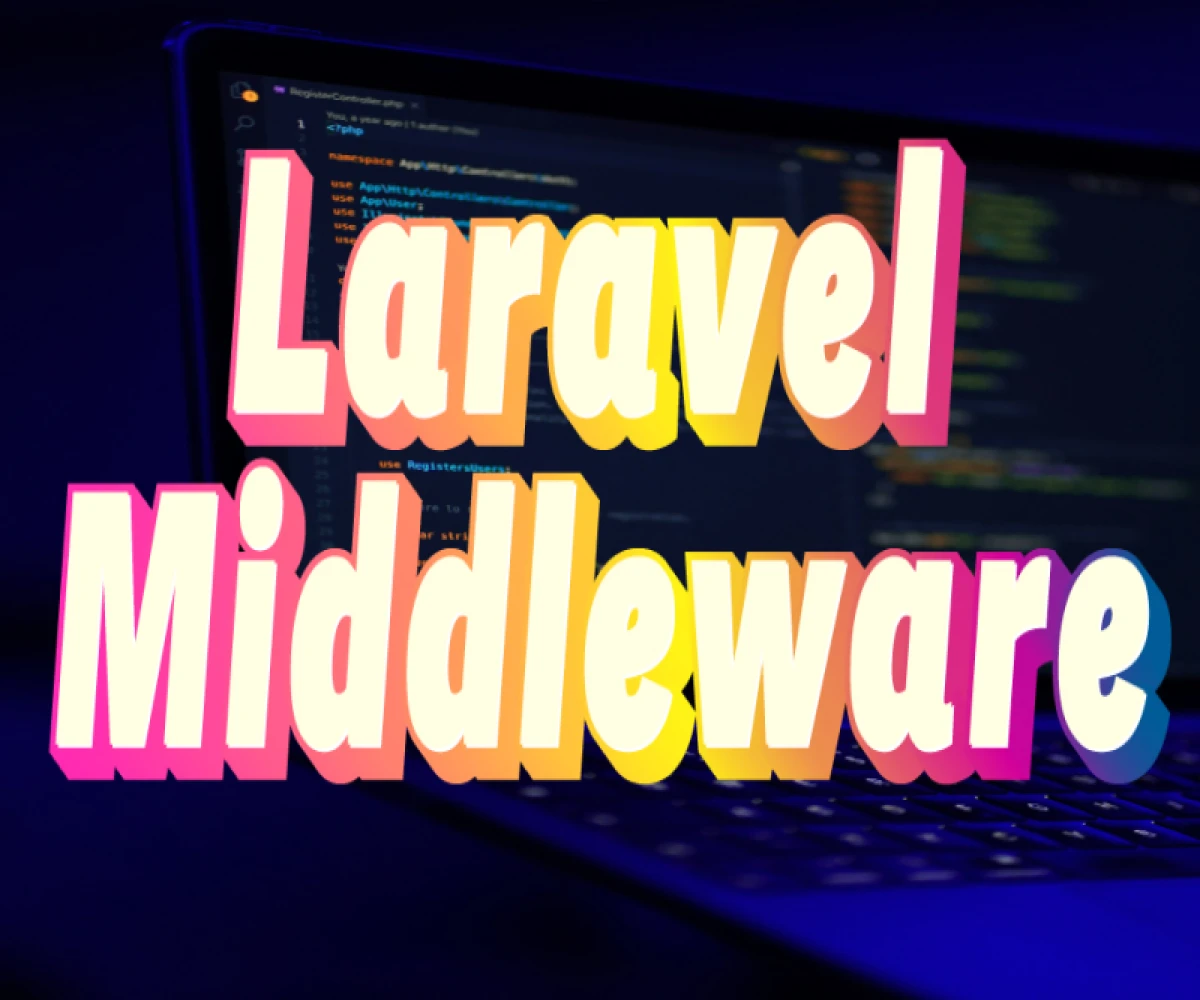
Unleash the Power of Laravel Middleware: Control Your App Like a Pro
Laravel Middleware: Interceptors for HTTP Requests and Responses
In Laravel, middleware acts as a powerful layer of control before HTTP requests reach your application's controllers. It provides a flexible mechanism for intercepting, inspecting, and potentially modifying incoming requests and outgoing responses. With middleware, you can achieve various functionalities, including:
- Authentication: Restrict access to specific routes based on user login status.
- Authorization: Enforce user permissions to control what actions they can perform.
- Logging: Record request details for auditing and troubleshooting purposes.
- Rate Limiting: Prevent abuse by throttling the number of requests allowed per user or IP address.
- CSRF Protection: Mitigate Cross-Site Request Forgery (CSRF) attacks.
- Input Validation: Ensure requests adhere to expected data formats and rules.
- Global Data Injection: Make common data like user information or site settings available throughout the application.
Creating Custom Middleware
-
Generate a Middleware Class: Utilize Laravel's Artisan command to generate a new middleware class:
Bash
php artisan make:middleware CheckAgeMiddleware
This creates a
CheckAgeMiddleware.php
file within theapp/Http/Middleware
directory. -
Implement the
handle
Method: The core logic of your middleware resides in thehandle
method. It receives an instance of the$request
object, a closure ($next
), and optionally additional arguments:PHP
<?php namespace App\Http\Middleware; use Closure; class CheckAgeMiddleware { public function handle($request, Closure $next, $age = 18) { // Logic to check user's age or retrieve it from the request if ($userAge < $age) { return redirect('/restricted'); // Or throw an exception } return $next($request); // Pass the request to the next middleware or controller } }
- The
$request
object provides access to request details like headers, parameters, cookies, etc. - The
$next
closure represents the next middleware in the chain or the final controller method to be executed. - You can optionally define additional arguments to customize your middleware's behavior.
- The
Registering Middleware
There are two primary ways to register middleware in Laravel:
-
Global Middleware: Register middleware classes in the
$middleware
property within theApp\Http\Kernel.php
file. This middleware will be applied to all incoming HTTP requests:PHP
protected $middleware = [ // ... other middleware App\Http\Middleware\CheckAgeMiddleware::class, ];
-
Route-Specific Middleware: Define middleware for specific routes or groups of routes using the
middleware
method when defining routes in yourroutes/web.php
orroutes/api.php
file:PHP
Route::get('/admin', function () { // Admin routes })->middleware('auth', 'admin'); // Apply both 'auth' and 'admin' middleware Route::group(['middleware' => 'age:21'], function () { // Routes requiring users to be 21 or older });
- The
middleware
method accepts a string containing the middleware class names separated by commas. - You can also pass arguments to middleware within parentheses, like
age:21
in the example above.
- The
Common Middleware Examples
-
Authentication Middleware (
auth
): This built-in middleware checks if a user is authenticated before proceeding. If not, it typically redirects to a login page. You can customize the behavior by overriding Laravel's default implementation. -
Authorization Middleware (
can
): This middleware verifies if the current user has the necessary permissions for a specific action. It requires theGate
service to define authorization logic.
Additional Considerations
- Middleware Stack: When multiple middleware are registered, they form a chain. Each middleware can modify the request or response before passing it to the next middleware or the controller.
- Terminating Middleware: Use the
$next->terminate($request, $response)
method within your middleware to stop the request processing and potentially return a custom response.
By effectively leveraging middleware in your Laravel application, you can streamline authentication, authorization, input validation, and other common tasks, enhancing your application's security, maintainability, and user experience.