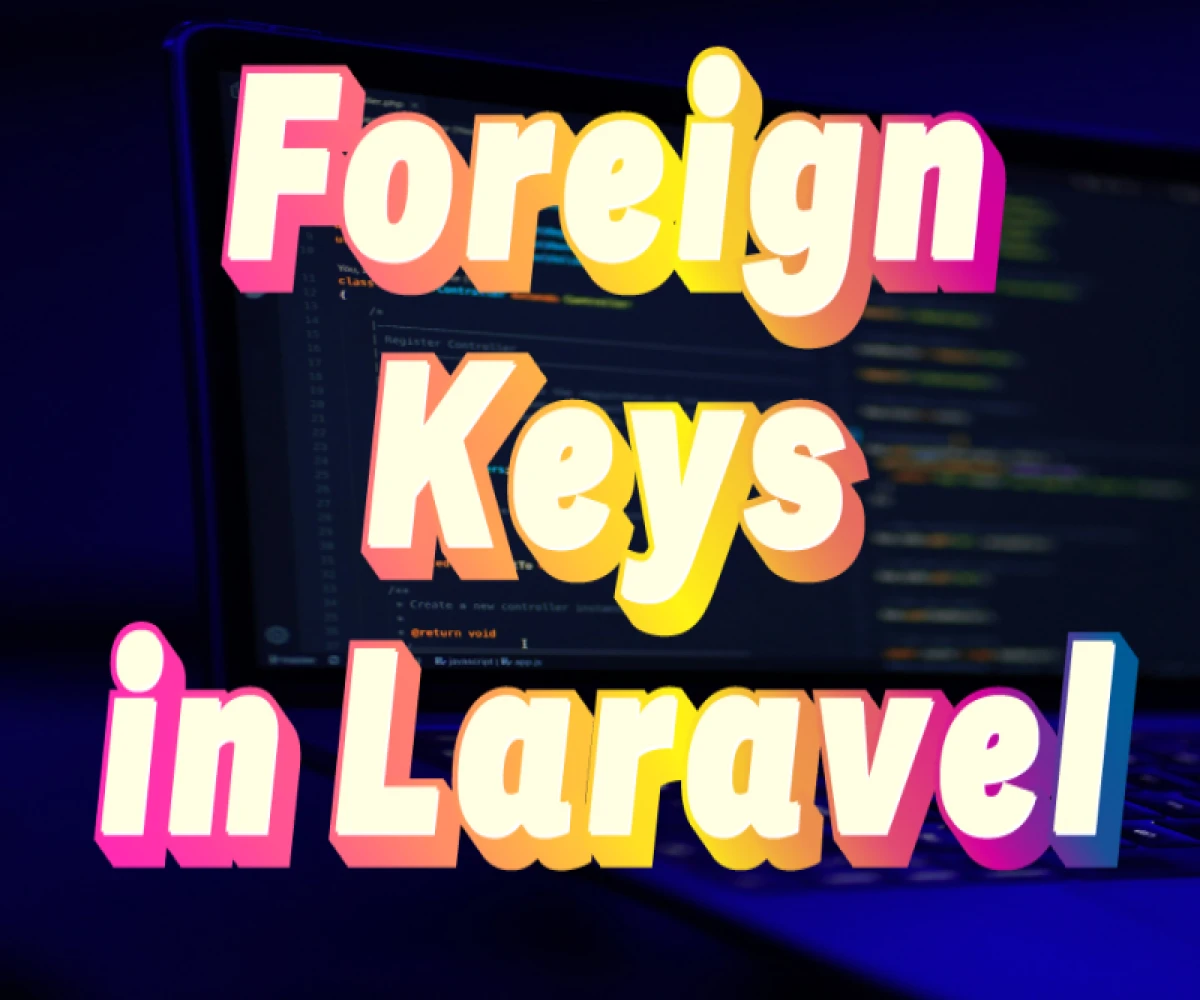
Advanced Techniques for Foreign Keys in Laravel
Understanding Foreign Keys in Laravel
Foreign keys are essential for establishing relationships between tables in your Laravel database. They ensure data consistency by referencing a primary key in another table. When a foreign key constraint is defined, Laravel enforces data integrity by preventing orphaned records.
Methods for Adding Foreign Keys in Laravel
There are several effective ways to implement foreign keys in Laravel migrations:
1. Traditional Method:
This method offers granular control over foreign key definitions:
PHP
Schema::table('products', function (Blueprint $table) {
$table->unsignedBigInteger('category_id');
$table->foreign('category_id')->references('id')->on('categories')
->onDelete('cascade')->onUpdate('cascade');
});
- Explanation:
$table->unsignedBigInteger('category_id');
creates an unsigned big integer column namedcategory_id
in theproducts
table.$table->foreign('category_id')->references('id')->on('categories');
defines the foreign key constraint, referencing theid
column in thecategories
table.->onDelete('cascade')->onUpdate('cascade');
specifies that when a category is deleted (ON DELETE), all related products referencing it will be deleted (CASCADE), and when a category's ID is updated (ON UPDATE), the corresponding foreign key values inproducts
will be updated (CASCADE).
2. foreignIdFor
Method (Laravel 7+):
This convenient method provides a streamlined approach:
PHP
Schema::table('products', function (Blueprint $table) {
$table->foreignIdFor(App\Models\Category::class)->constrained();
});
- Explanation:
$table->foreignIdFor(App\Models\Category::class);
creates a foreign key column namedcategory_id
(following Laravel's convention) with the appropriate data type based on theCategory
model's primary key. It references theid
column in thecategories
table.->constrained();
automatically adds the foreign key constraint with defaultonDelete
andonUpdate
behaviors (usuallySET NULL
orRESTRICT
).
3. Customizing foreignIdFor
:
You can customize the column name and constraint options:
PHP
Schema::table('products', function (Blueprint $table) {
$table->foreignIdFor(App\Models\Category::class, 'custom_category_id')
->constrained('categories', 'custom_constraint_name')
->onDelete('cascade')
->onUpdate('cascade');
});
- Explanation:
$table->foreignIdFor(App\Models\Category::class, 'custom_category_id');
creates a foreign key column namedcustom_category_id
.->constrained('categories', 'custom_constraint_name');
specifies the foreign table and constraint name.->onDelete('cascade')->onUpdate('cascade');
sets customonDelete
andonUpdate
actions.
Choosing the Right Method
- The traditional method provides the most fine-grained control.
- Use
foreignIdFor
for convenience and Laravel's conventions. - Customize
foreignIdFor
when you need specific column names or constraint behaviors.
Additional Considerations
- Existing Tables: To add foreign keys to existing tables, use the
alterTable
method in a migration:
PHP
Schema::table('products', function (Blueprint $table) {
$table->foreignIdFor(App\Models\Category::class)->constrained();
});
- Referential Integrity: Ensure the referenced table and column exist before creating the foreign key.
- Cascade vs. Set Null vs. Restrict: Choose the appropriate
onDelete
andonUpdate
actions based on your data relationships and integrity requirements. - Testing: Thoroughly test your foreign key constraints to verify data consistency.
By following these guidelines, you'll effectively establish relationships between your Laravel tables using foreign keys, enhancing data integrity and managing your database relationships efficiently.