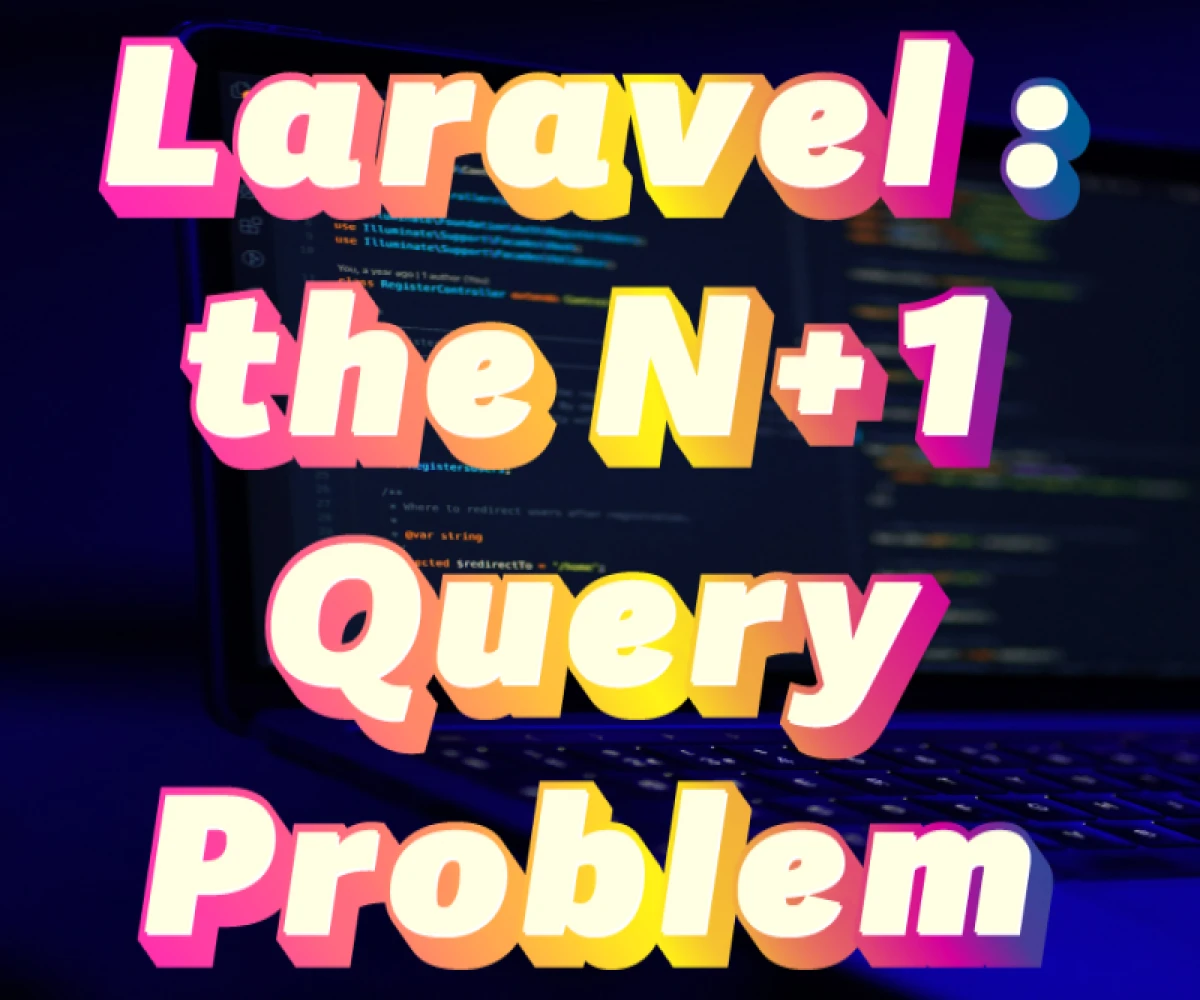
What is the N+1 Problem in Laravel ?
Understanding the N+1 Query Problem
In Laravel applications, the N+1 query problem arises when you unintentionally execute multiple database queries to fetch related model data. This occurs because Eloquent's default behavior is lazy loading, where it retrieves data for related models only when you access their properties.
Here's a simplified example:
PHP
// Posts controller
$posts = Post::all();
foreach ($posts as $post) {
echo $post->title . ' by ' . $post->user->name; // This triggers an additional query for each user
}
In this scenario, for N
posts, you'll end up with N + 1
queries:
- One query to fetch all posts.
N
additional queries to fetch the user for each post (one per post).
This can significantly impact performance, especially when dealing with large datasets.
Eager Loading to the Rescue
Eager loading allows you to fetch related model data in a single database query, reducing the number of queries to just one. Here's how to implement it:
PHP
$posts = Post::with('user')->get();
foreach ($posts as $post) {
echo $post->title . ' by ' . $post->user->name; // User data is already available, no additional query
}
In this example:
- A single query is executed to fetch all posts along with their associated user data.
- Within the loop, you can access
$post->user->name
without triggering extra queries because the user data is already loaded.
Key Points about Eager Loading:
- Reduced Database Calls: Eager loading significantly improves performance by minimizing database interactions.
- Increased Memory Usage: Loading more data at once might consume more memory, especially with large datasets.
- Selective Loading: You can specify which relationships to eager load using the
with()
method:
PHP
$posts = Post::with('user', 'comments')->get(); // Eager load user and comments
- Lazy Loading vs. Eager Loading: Use eager loading when you know you'll need related model data in your application logic or views. For data that might not be required, consider lazy loading (the default behavior).
Additional Tips
- Profiling: Use Laravel's debugging tools (like Debugbar) to identify N+1 query problems and profile the performance impact of eager loading.
- Trade-offs: Consider the trade-off between database calls and memory usage when deciding between eager and lazy loading.
- Pagination: If you're working with large datasets, use pagination to break down data into smaller chunks and avoid loading everything at once.
By effectively using eager loading, you can optimize your Laravel application's performance and ensure a smooth user experience.