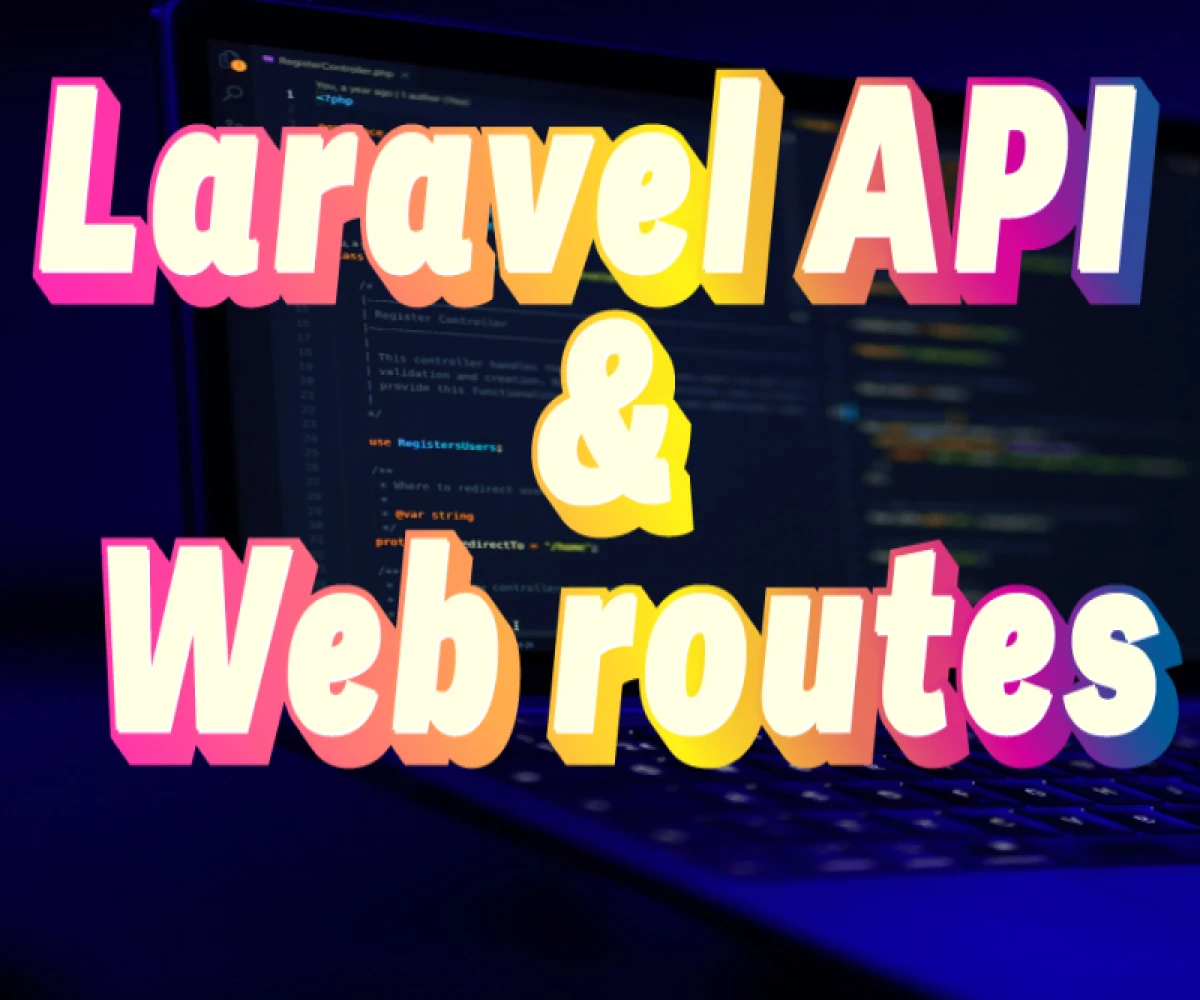
What is the difference between Laravel API and Web routes?
Laravel
4 months ago
Laravel API vs Web Routes: A Comprehensive Guide
Laravel, a robust PHP framework, offers two primary types of routes: API and Web. While they might seem similar at first glance, they serve distinct purposes and cater to different application needs.
Understanding Web Routes
Web routes are primarily designed for traditional web applications that interact directly with users. These routes are characterized by:
- Purpose: Handling user interactions like login, registration, form submissions, and displaying content.
- Middleware: Automatically includes session state, CSRF protection, and other web-specific middleware for enhanced security.
- Output: Typically renders HTML views using the Blade templating engine.
- Use Cases: Perfect for building user interfaces, dashboards, and any application where you need to interact with users directly.
Demystifying API Routes
API routes, on the other hand, are built specifically for creating stateless APIs that interact with other applications or services. Key characteristics include:
- Purpose: Exchanging data with external clients like mobile apps, single-page applications (SPAs), or other systems.
- Middleware: Uses a different middleware group by default, often excluding session and CSRF protection to optimize performance.
- Output: Primarily returns JSON or other structured data formats for easy consumption by external applications.
- Use Cases: Ideal for building RESTful APIs, microservices, and any application that needs to share data with other systems.
Key Differences Summarized
Feature | Web Routes | API Routes |
---|---|---|
Purpose | User interface | Data exchange |
Middleware | Session, CSRF, etc. | Often minimal |
Output | Views (HTML) | JSON, XML, etc. |
Statefulness | Stateful | Stateless |
When to Use Which?
- Web Routes: For user-facing features like login, registration, profile management, and content display.
- API Routes: For providing data to external clients, such as mobile apps, single-page applications, or other services.
Example
PHP
// routes/web.php
Route::get('/', function () {
return view('welcome');
});
// routes/api.php
Route::get('/users', function () {
return User::all();
});
Additional Considerations
- Route Groups: Organize your routes effectively using
Route::group()
. - Route Parameters: Utilize parameters for dynamic URL segments in both types of routes.
- Route Names: Improve readability and maintainability by assigning names to routes.
- Route Closures: Define route logic directly within the route closure for convenience.
By understanding the fundamental differences between Laravel's web and API routes, you can make informed decisions about their usage in your projects. This knowledge will help you build well-structured, efficient, and scalable applications.