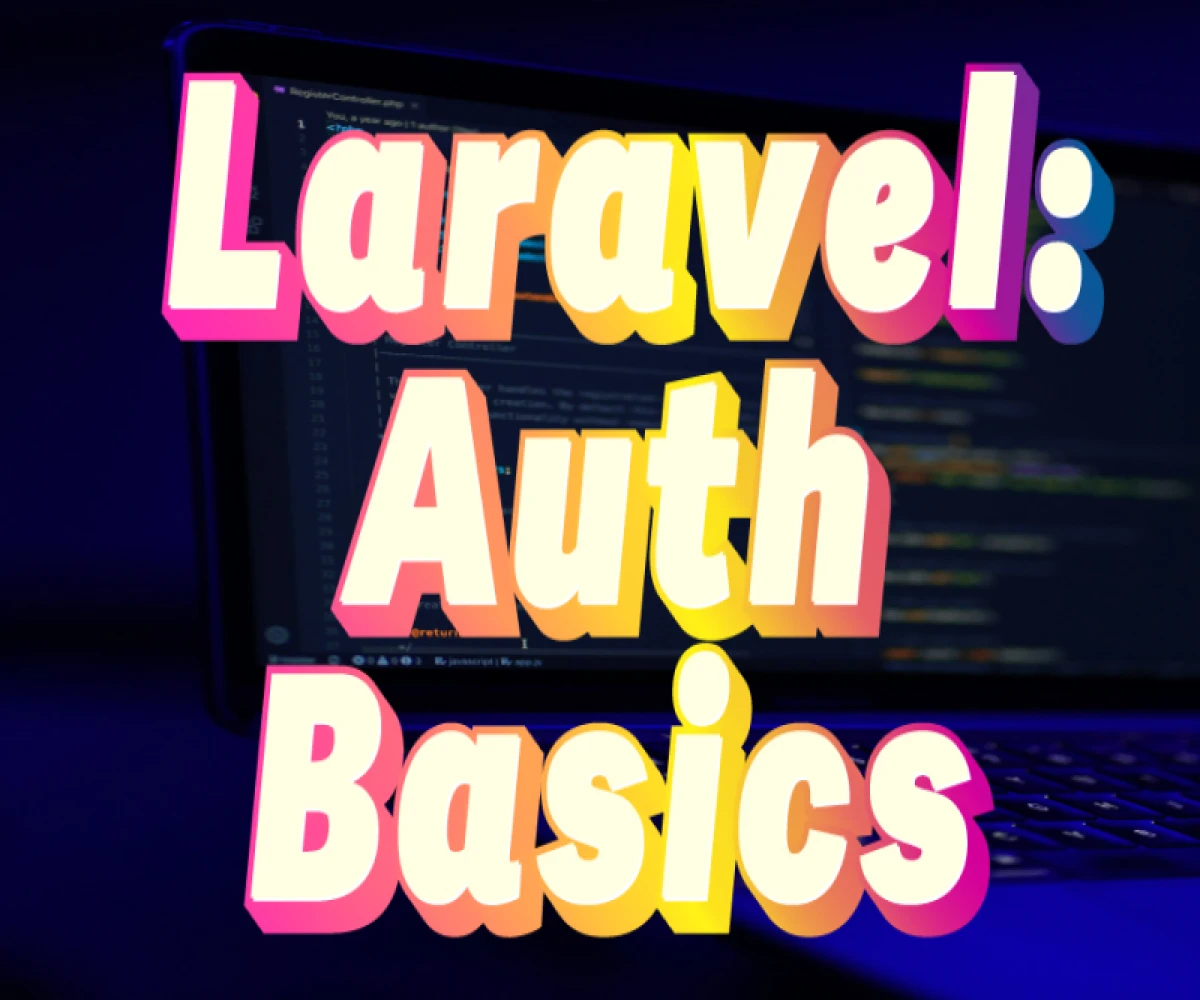
Mastering Laravel User Authentication
Laravel Authentication Basics Tutorial
This tutorial guides you through the fundamentals of authentication in Laravel, covering starter kits, accessing the default authentication model, implementing checks in controllers and Blade templates, and utilizing authentication middleware.
Prerequisites:
- Basic understanding of PHP and Laravel concepts
- A Laravel project set up (https://laravel.com/docs/11.x/installation)
1. Starter Kit: Laravel UI (Bootstrap)
For a quick and convenient setup with Bootstrap styling, Laravel offers the laravel/ui
package. Install it using Composer:
Bash
composer require laravel/ui
Next, publish the necessary assets and configuration:
Bash
php artisan ui vue --auth # For Vue.js frontend (optional)
php artisan ui bootstrap # For Bootstrap frontend
This generates controllers, views, and migrations for user authentication functionalities.
2. Default Auth Model and Accessing Fields
Laravel provides a default user model (App\Models\User
). You can access its fields like any Eloquent model:
PHP
$user = Auth::user();
$name = $user->name;
$email = $user->email;
3. Checking Authentication in Controllers and Blade Templates
Controllers:
PHP
use Illuminate\Support\Facades\Auth;
public function someMethod()
{
if (Auth::check()) {
// User is authenticated
$user = Auth::user();
// ...
} else {
// User is not authenticated
return redirect('/login');
}
}
Blade Templates:
HTML
@if (Auth::check())
<p>Welcome, {{ Auth::user()->name }}!</p>
<a href="{{ route('logout') }}">Logout</a>
@else
<a href="{{ route('login') }}">Login</a>
<a href="{{ route('register') }}">Register</a>
@endif
4. Authentication Middleware
Middleware allows you to control access to routes based on authentication status. Create a middleware:
Bash
php artisan make:middleware AuthMiddleware
In the middleware (app/Http/Middleware/AuthMiddleware.php
):
PHP
public function handle($request, Closure $next)
{
if (!Auth::check()) {
return redirect('/login');
}
return $next($request);
}
Register the middleware in app/Http/Kernel.php
:
PHP
protected $routeMiddleware = [
'auth' => \App\Http\Middleware\AuthMiddleware::class,
];
Apply middleware to routes:
PHP
Route::get('/profile', function () {
// This route requires authentication
})->middleware('auth');
Additional Notes:
- Remember to configure your email settings in
.env
for features like password reset. - Laravel provides further authentication functionalities like two-factor authentication and social login integration, which you can explore in the documentation (https://laravel.com/docs/11.x/authentication).
- Consider using a more robust starter kit like Laravel Jetstream for a broader set of features and modern UI components (https://jetstream.laravel.com/).
By following these steps, you'll have a solid foundation for user authentication in your Laravel application, using starter kits for convenience, accessing user data effectively, and implementing authentication checks in controllers and Blade templates to control access and tailor the user experience.