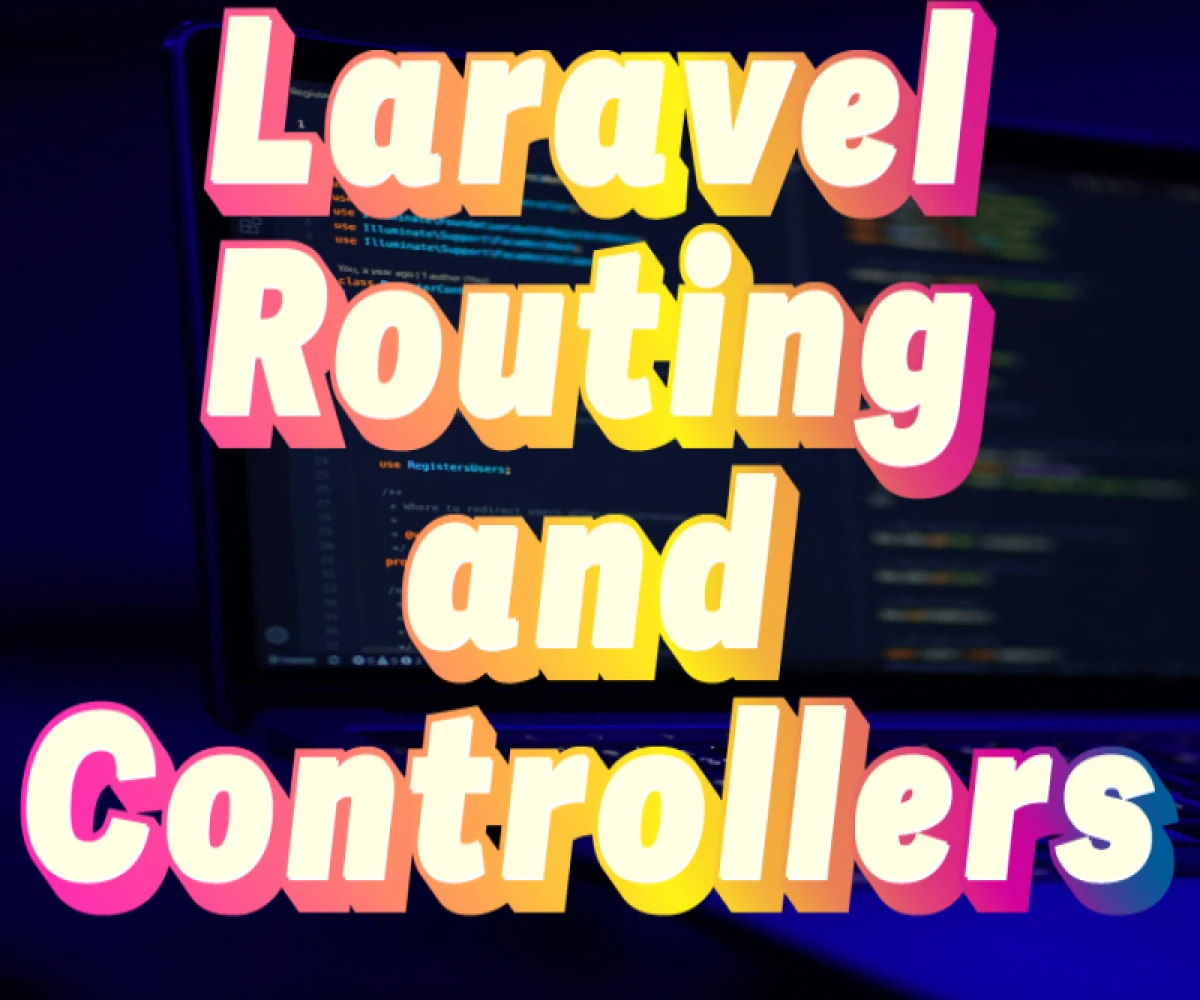
Laravel: Navigate Your App Like a Pro with Routing and Controllers
Laravel
4 months ago
Understanding Routing and Controllers in Laravel
- Routing: In Laravel, routing establishes a map between URLs (web addresses) and the corresponding logic in your application that handles those requests. When a user visits a specific URL in your Laravel application, the routing system directs the request to the appropriate controller method for processing.
- Controllers: Controllers are classes that encapsulate the business logic of your application. They contain methods (functions) that handle incoming requests, retrieve data from the database or other sources, perform calculations, and ultimately generate a response (usually a view) to be sent back to the user's browser.
Creating Your First Route and Controller
-
Generate a Controller (Optional):
- If you don't have a controller yet, you can use the Artisan command to create a new one:
Bash
php artisan make:controller WelcomeController
- This creates a
WelcomeController.php
file in theapp/Http/Controllers
directory.
- If you don't have a controller yet, you can use the Artisan command to create a new one:
-
Define the Route:
- Open the
routes/web.php
file. This file contains all the routes for your web application. - Add a route to define the URL pattern and the controller method that handles it:
PHP
Route::get('/', 'WelcomeController@index');
- Explanation:
Route::get()
: This method defines a route that handles GET requests (the most common type of request in web applications).'/'
: This specifies the URL pattern. In this case, the route matches the root URL of your application (usuallyhttp://your-app.com/
).'WelcomeController@index'
: This tells Laravel to call theindex
method of theWelcomeController
class when this route is matched. The@
symbol separates the controller class from the method name.
- Open the
-
Implement the Controller Method:
- Open the
WelcomeController.php
file and add theindex
method:PHP
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; class WelcomeController extends Controller { public function index(Request $request) { // Your application logic here // You can access the request object to get information about the request // (e.g., user input, headers) return view('welcome'); // Return the 'welcome' view } }
- Explanation:
- The
index
method takes anIlluminate\Http\Request
object as an argument, which provides information about the incoming request (optional). - Inside the method, you can write your application logic, interact with the database, perform calculations, etc.
- In this example, the method simply returns the
'welcome'
view, which tells Laravel to render the corresponding blade template file (resources/views/welcome.blade.php
).
- The
- Open the
Running Your Application
- Start the Development Server:
- Run the following command in your terminal to start the built-in Laravel development server:
Bash
php artisan serve
- Run the following command in your terminal to start the built-in Laravel development server:
- Access Your Application:
- Open a web browser and visit
http://localhost:8000/
(the default port for the development server). You should see the content of yourwelcome
view.
- Open a web browser and visit
Additional Considerations
- Controller Methods: Controllers can have multiple methods to handle different types of requests (GET, POST, PUT, DELETE) for the same URL.
- Views: Views are Blade templates (
.blade.php
files) that define the HTML structure of your application's pages. They can contain dynamic content based on data passed from the controller. - Request Object: The
Request
object provides detailed information about the incoming request, such as user input, headers, cookies, etc. - More Complex Routing: Laravel supports more complex route definitions with parameters, middleware (for authentication, authorization, etc.), and other features as your application grows.
Learning More:
For a deeper understanding of Laravel Routing and Controllers, refer to the official Laravel documentation: https://laravel.com/docs/11.x/routing