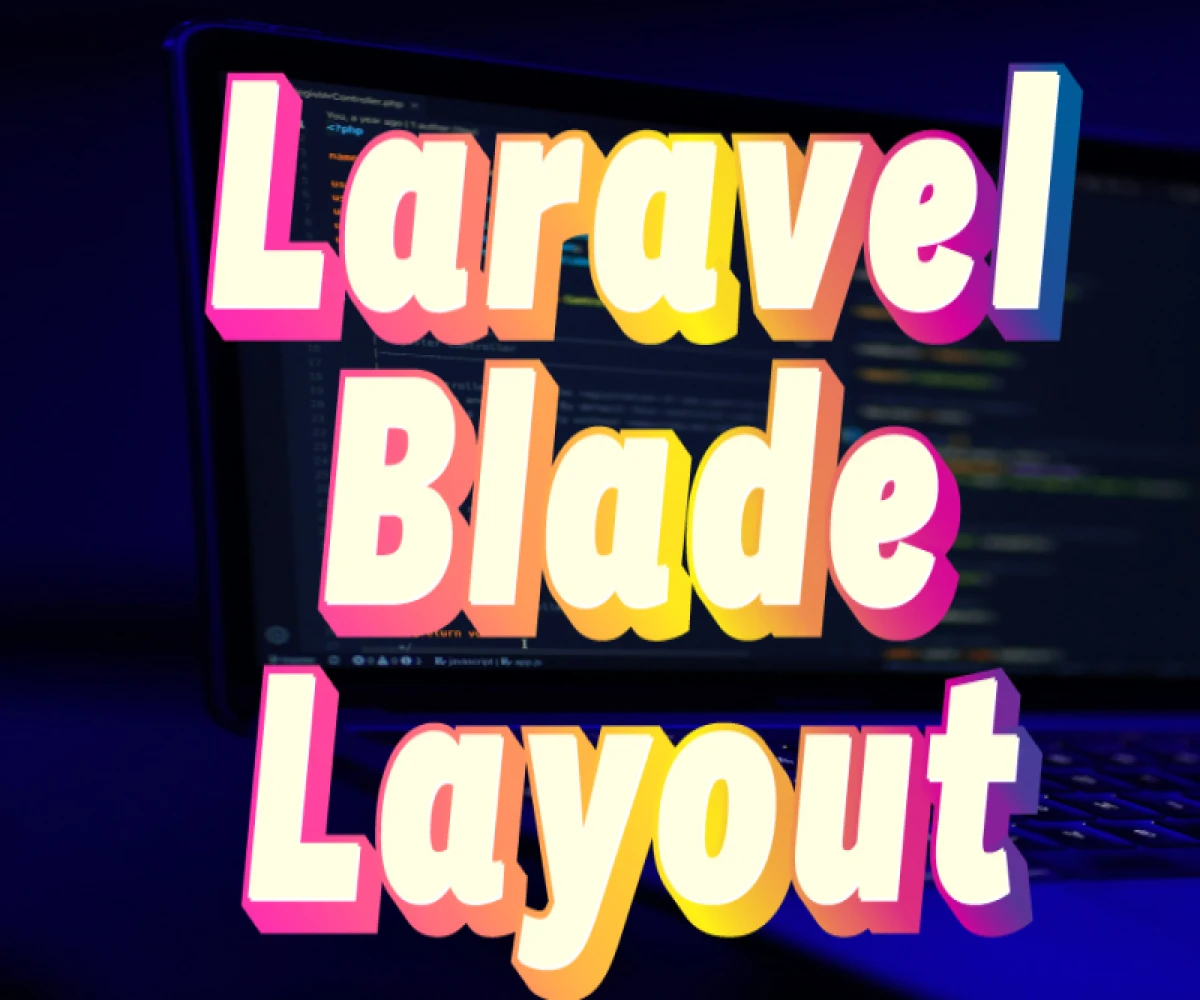
How To Create a Laravel Blade Layout Templating ?
Understanding Layouts in Laravel
In Laravel, layouts (often called master pages) provide a reusable structure for your application's views. They define the common elements like header, navigation, footer, and sidebars, while individual views (like home, about, contact) focus on their specific content. This separation promotes code maintainability and consistency across your application.
Creating the Layout File
- Navigate to Views: Using your terminal, navigate to the
resources/views/layouts
directory in your Laravel project. This directory is specifically dedicated to layout files. - Create a New File: Create a new Blade template file, typically named
default.blade.php
or something similar that reflects your application's style. This file will house the layout structure.
Building the Layout Structure
- HTML Structure: Start by adding the basic HTML structure, including the
<!DOCTYPE html>
,<html>
,<head>
, and<body>
tags. - Head Section: Within the
<head>
section, define essential elements like<title>
, meta tags for character encoding and description, and any necessary CSS links to style your layout. - Header: Create a header section using appropriate HTML elements (e.g.,
<header>
,<nav>
,<ul>
) to hold your application's logo, navigation links, or other branding elements. - Content Section: Introduce a section where individual views will insert their content. This is typically achieved using the Blade
@yield('content')
directive. The@yield
directive acts as a placeholder where content from child views will be injected. - Sidebar (Optional): If your layout incorporates a sidebar, create a dedicated section using HTML elements like
<aside>
to hold sidebar content. - Footer: Define a footer section using
<footer>
or other suitable elements to display copyright information, social media links, or any additional content you want to appear consistently across all pages.
Example Layout (default.blade.php
):
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>{{ config('app.name') }}</title>
<link rel="stylesheet" href="{{ asset('css/app.css') }}">
</head>
<body>
<header>
</header>
<main>
@yield('content')
</main>
<aside>
</aside>
<footer>
</footer>
<script src="{{ asset('js/app.js') }}"></script>
</body>
</html>
Creating Individual Views
- Navigate to Views: Go to the appropriate directory within your
resources/views
directory (e.g.,home
,about
,contact
) to create individual views for different sections of your application. - Create View Files: Create Blade template files (e.g.,
home.blade.php
,about.blade.php
,contact.blade.php
) for each view. - Extend Layout: In each view file, use the
@extends('layouts.default')
directive to indicate that the view inherits the structure from thedefault.blade.php
layout file. - Define Content: Within the individual views, define the specific content that will be displayed within the
@yield('content')
section of the layout.
Example View (home.blade.php
):
HTML
@extends('layouts.default')
@section('content')
<h1>Welcome to the Home Page!</h1>
<p>This is the content of the home page.</p>
@endsection
Routing (Optional):
While layout creation doesn't necessarily require route definitions, if you have routes set up, you might want to adjust them to render the created layout. For example, in your routes/web.php
file:
PHP
Route::get('/', function () {
return view('home'); // Assuming you have a home route
});
Additional Tips
- Component-Based Layouts (Laravel 9+): Consider using Laravel's component system for more complex layouts or reusable UI elements.
- Blade Syntax: Explore Laravel's Blade templating engine for conditional statements,loops and other features that improve the structure and logic of your views.