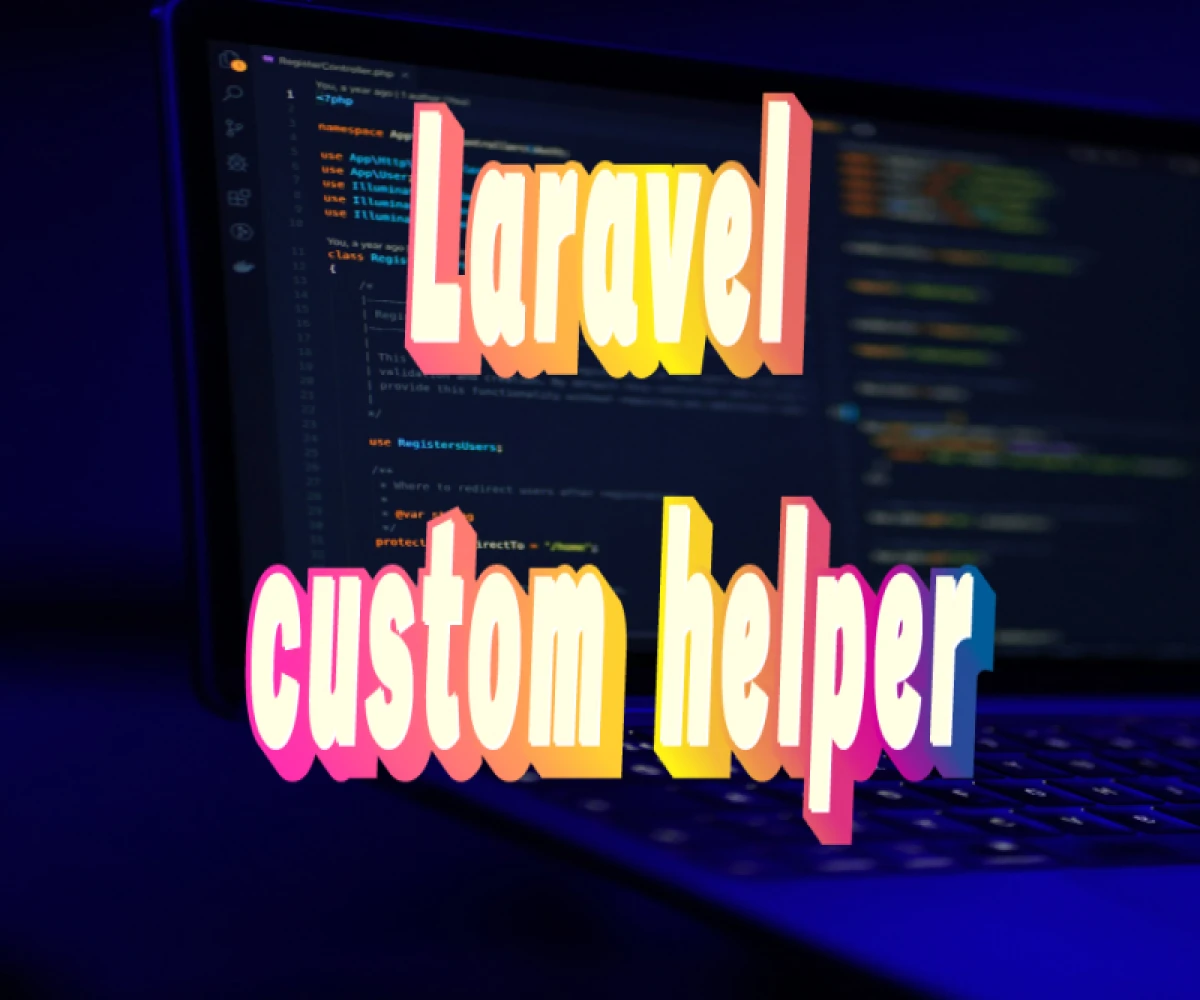
How to add a helper function in Laravel ?
Introduction:
In this tutorial, we'll explore how to create and utilize custom helper files in your Laravel applications. Helper files are invaluable for encapsulating reusable code, improving code organization, and enhancing maintainability.
Step 1: Creating the Helper File
- Create a Directory: Begin by creating a new directory named
Helpers
within yourapp
directory. If the directory already exists, proceed to the next step. - Create the Helper File: Within the
Helpers
directory, create a new PHP file. Name it according to its purpose, such asStringHelper.php
,ArrayHelper.php
, orGeneralHelper.php
.
Step 2: Defining Helper Functions
- Open the Helper File: Open the newly created PHP file in your preferred code editor.
- Define Functions: Inside the file, define PHP functions that encapsulate common tasks or logic that you frequently use throughout your application.
Example:
PHP
<?php
if (! function_exists('str_to_slug')) {
function str_to_slug($string) {
// Implementation for converting a string to slug format
// (e.g., using the Illuminate/Support/Str facade)
return Str::slug($string);
}
}
if (! function_exists('array_flatten')) {
function array_flatten($array) {
// Implementation for flattening a multidimensional array
return array_merge([], ...$array);
}
}
?>
Step 3: Registering the Helper File (Optional)
- Update
composer.json
: If you want to make your helper functions globally accessible, open yourcomposer.json
file and add the following under theautoload
section:
JSON
"autoload": {
"files": [
"app/Helpers/StringHelper.php",
"app/Helpers/ArrayHelper.php"
]
}
-
Update Autoloads: After modifying
Bashcomposer.json
, run the following command in your terminal to update the autoloader:composer dump-autoload
Step 4: Using Helper Functions
- Access Helper Functions: You can now use your helper functions directly within your controllers, models, views, or any other part of your Laravel application.
Example:
PHP
use App\Helpers\StringHelper;
class MyController extends Controller
{
public function index()
{
$slug = StringHelper::str_to_slug('My Awesome Title');
// ...
}
}
Additional Tips:
- Namespaces: Utilize namespaces for better organization if you have many helper functions.
- Helper Classes: For more complex logic, consider creating dedicated helper classes instead of using simple functions.
- Testing: Thoroughly test your helper functions to ensure they work as expected.
Conclusion:
By following these steps, you can effectively create and utilize custom helper files in your Laravel projects. This approach enhances code reusability, improves maintainability, and promotes a more organized and efficient development workflow.
I hope this blog tutorial proves helpful!
Note: This tutorial assumes basic familiarity with Laravel and its directory structure.
Disclaimer: This is a basic example. The actual implementation of helper functions will depend on your specific project requirements.