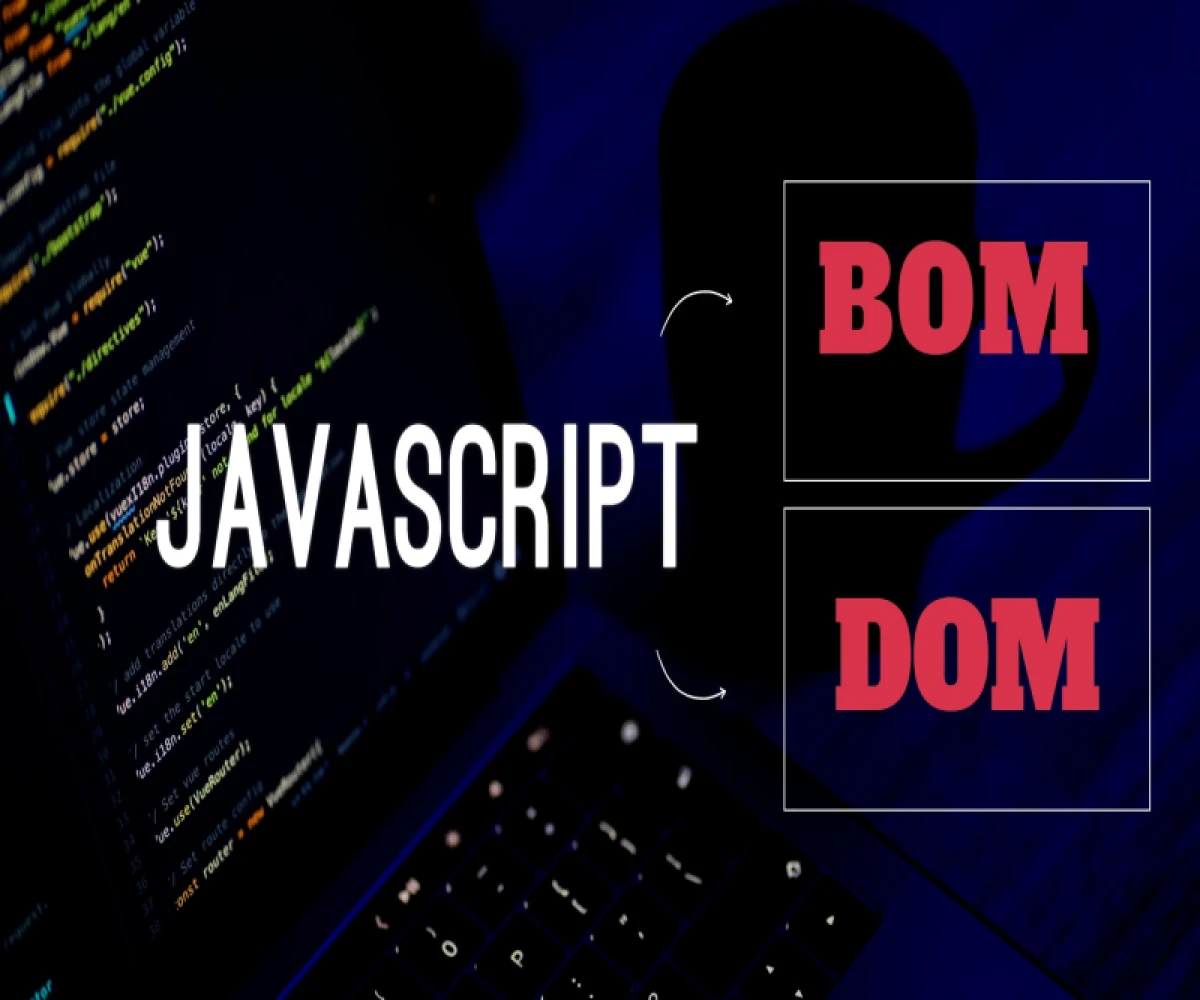
A Beginner's Guide to BOM and DOM in JavaScript
Introduction:
JavaScript, the language that powers the dynamic aspects of web development, provides two essential components for interacting with web pages: the Browser Object Model (BOM) and the Document Object Model (DOM). In this tutorial, we'll explore the fundamentals of BOM and DOM, understanding how they work and how you can leverage them to enhance your web development skills.
Browser Object Model (BOM):
1. What is BOM?
The Browser Object Model (BOM) is a JavaScript API that allows interaction with the browser itself rather than the content of a web page. It provides objects and methods for controlling browser behavior, managing windows, and handling navigation.
2. Common BOM Components:
- Window Object:
The `window` object represents the browser window and serves as the root object for the BOM. It includes properties like `location`, `navigator`, and methods like `alert()`.
// Example: Accessing the current URL
let currentURL = window.location.href;
- Navigator Object:
The `navigator` object provides information about the browser, such as its name and version.
// Example: Getting the browser name
let browserName = window.navigator.appName;
-Screen Object:
The `screen` object contains information about the user's screen, such as width and height.
// Example: Getting screen width
let screenWidth = window.screen.width;
Document Object Model (DOM):
1. What is DOM?
The Document Object Model (DOM) is a programming interface for web documents. It represents the structure of a document as a tree of objects, allowing scripts to dynamically update the content, structure, and style of a document.
2. Common DOM Concepts:
- Document Object:
The `document` object is the main entry point to the DOM and represents the entire HTML document.
// Example: Changing the content of an element
document.getElementById("example").innerHTML = "New Content";
- Element Objects:
Elements on a web page, such as paragraphs or images, are represented as element objects. You can manipulate their attributes and content using JavaScript.
// Example: Changing the style of an element
let element = document.getElementById("example");
element.style.color = "blue";
- Event Handling:
DOM allows you to respond to user interactions (events) such as clicks or keypresses. Event listeners can be added to elements.
// Example: Adding a click event listener
document.getElementById("exampleButton").addEventListener("click", function() {
alert("Button Clicked!");
});
Conclusion:
Understanding the Browser Object Model (BOM) and Document Object Model (DOM) is crucial for effective web development. The BOM provides control over the browser, while the DOM enables dynamic manipulation of web page content. By mastering these concepts, you can create more interactive and responsive web applications.