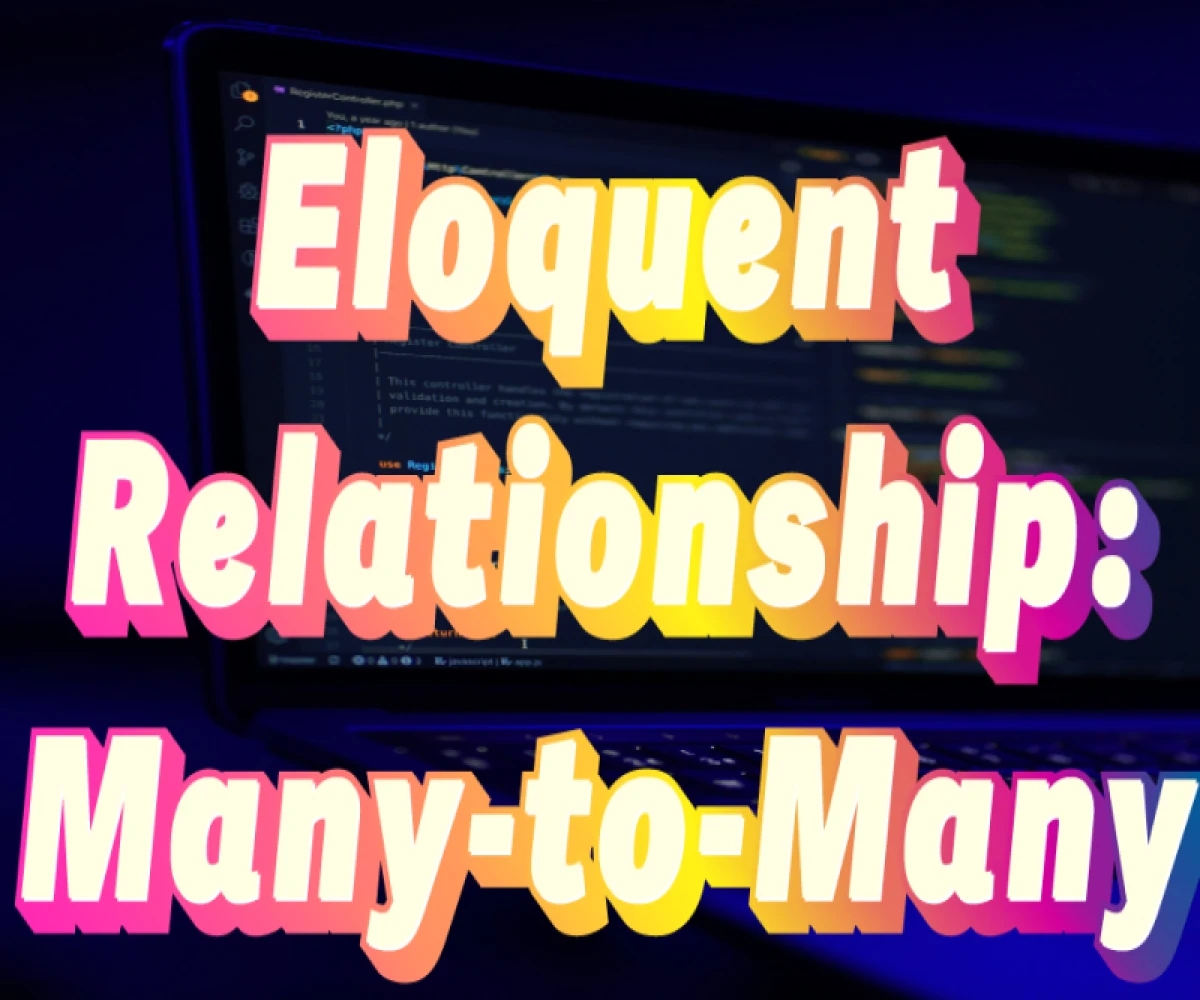
Laravel Many to Many relationship with example
Understanding belongsToMany in Laravel
The belongsToMany
relationship in Laravel's Eloquent ORM facilitates modeling many-to-many relationships between database tables. This means a single record in one table can be associated with multiple records in another table, and vice versa.
Example Scenario: Blog Posts and Tags
Let's consider a common example: blog posts and tags. A blog post can have multiple tags, and a tag can be associated with multiple posts. Here's how we can model this relationship in Laravel:
1. Database Schema:
We'll need three tables:
posts
: Contains information about blog posts (id, title, content, etc.)tags
: Holds details about tags (id, name, description, etc.)post_tag
(pivot table): This table links posts and tags. It typically has two foreign keys:post_id
(referencing theposts.id
) andtag_id
(referencing thetags.id
). Optionally, it can store additional data specific to the relationship, such as a timestamp or a custom order.
2. Model Definitions:
Create model classes for Post
and Tag
:
PHP
// app/Models/Post.php
<?php
namespace App\Models;
use Illuminate\Database\Eloquent\Model;
use Illuminate\Database\Eloquent\Relations\BelongsToMany;
class Post extends Model
{
public function tags(): BelongsToMany
{
return $this->belongsToMany(Tag::class, 'post_tag');
}
}
// app/Models/Tag.php
<?php
namespace App\Models;
use Illuminate\Database\Eloquent\Model;
use Illuminate\Database\Eloquent\Relations\BelongsToMany;
class Tag extends Model
{
public function posts(): BelongsToMany
{
return $this->belongsToMany(Post::class, 'post_tag');
}
}
Explanation:
- In the
Post
model, thetags()
method defines abelongsToMany
relationship with theTag
model. The second argument ('post_tag'
) specifies the name of the pivot table. - Similarly, the
Tag
model defines aposts()
method to access the related posts.
3. Attaching and Detaching Tags:
-
To attach a tag to a post:
PHP
$post = Post::find(1); $tag = Tag::find(2); $post->tags()->attach($tag->id);
-
To detach a tag from a post:
PHP
$post = Post::find(1); $tag = Tag::find(2); $post->tags()->detach($tag->id);
-
To attach multiple tags at once:
PHP
$post = Post::find(1); $tagIds = [1, 2, 3]; $post->tags()->attach($tagIds);
4. Accessing Related Tags:
-
Retrieve all tags associated with a post:
PHP
$post = Post::find(1); $tags = $post->tags; // Collection of Tag models
-
Iterate through the tags:
PHP
foreach ($post->tags as $tag) { echo $tag->name; // Access tag attributes }
5. Eager Loading and Pivot Data:
-
For performance optimization, use eager loading to retrieve related tags along with the post in a single query:
PHP
$post = Post::with('tags')->find(1);
-
To access pivot table data (if it contains additional columns):
PHP
foreach ($post->tags as $tag) { echo $tag->pivot->created_at; // Access pivot attributes }
Additional Notes:
-
Customize the pivot table name by passing a third argument to the
belongsToMany
method:PHP
$this->belongsToMany(Tag::class, 'post_tag', 'post_tag_meta');
-
Define custom attributes for the pivot table using the
withPivot
method:PHP
public function tags(): BelongsToMany { return $this->belongsToMany(Tag::class, 'post_tag') ->withPivot('created_by', 'approved'); }
-
6. Detaching All Tags or Specific Conditions:
-
To detach all tags from a post:
PHP
$post = Post::find(1); $post->tags()->detach();
-
To detach tags based on specific conditions:
PHP
$post = Post::find(1); $post->tags()->detach(function ($query) { $query->where('name', 'like', '%tech%'); // Detach tags containing "tech" });
-
7. Syncing Tags:
The
sync
method allows you to completely replace the existing tags associated with a post:PHP
$post = Post::find(1); $newTagIds = [2, 4, 5]; $post->tags()->sync($newTagIds);
8. Updating Pivot Data:
If your pivot table stores additional data, you can update it while attaching or syncing tags:
PHP
$post = Post::find(1); $tagWithPivotData = [ 'tag_id' => 3, 'approved' => true, ]; $post->tags()->attach($tagWithPivotData);
9. Validation and Business Logic:
- Implement validation rules to ensure valid data is attached to the relationship (e.g., maximum number of tags per post).
- Use Laravel's events or custom logic to handle actions triggered by attaching or detaching tags (e.g., updating tag usage statistics, sending notifications).
- Choose appropriate column names and data types for your pivot table based on the specific relationship requirements.
- Consider using migration files to manage the schema changes for the pivot table.
- For large datasets, explore techniques like lazy loading or chunk detaching to improve performance.
-
By effectively utilizing the
belongsToMany
relationship in Laravel, you can efficiently manage many-to-many associations within your application, improving data organization and enhancing user experience.10. Polymorphic Relationships:
Laravel's
belongsToMany
can also model polymorphic relationships, where a model can have a many-to-many relationship with multiple other models. This requires additional configuration, but provides flexibility for more complex scenarios. Refer to the Laravel documentation for details on polymorphic relationships https://laravel.com/docs/11.x/eloquent-relationships