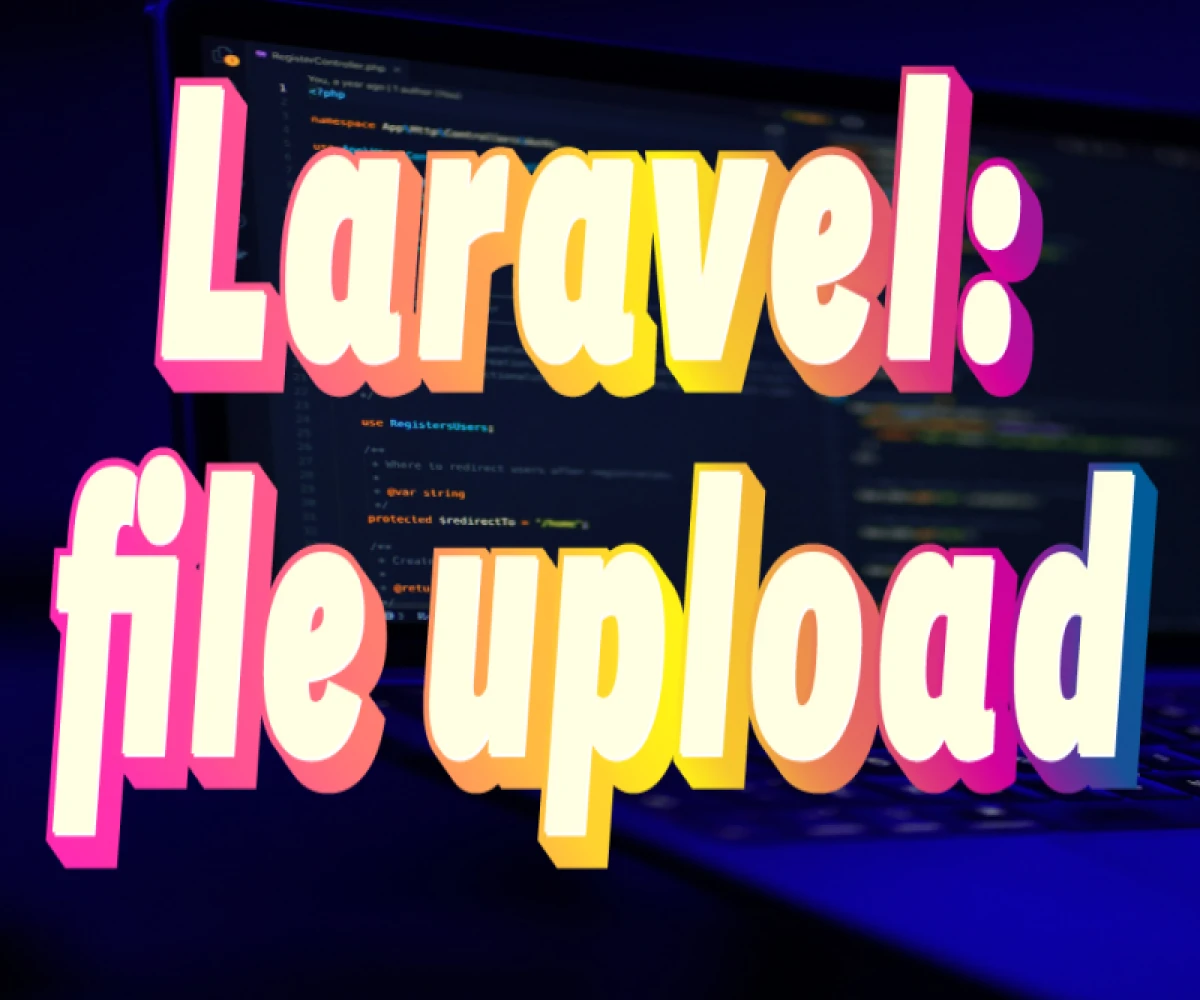
How to upload a file in Laravel ?
Uploading Files and Images in Laravel
Introduction
Laravel provides a streamlined approach to handling file uploads. This tutorial will guide you through the essential steps to successfully upload and manage files in your Laravel application.
Prerequisites
- A Laravel project set up.
- Basic understanding of Laravel routing, controllers, and views.
Step-by-Step Guide
1. Configure File Storage
Laravel utilizes the storage
directory to store uploaded files. Ensure this directory is configured correctly. You can check the filesystem
disk configuration in config/filesystems.php
.
2. Create a Model and Migration (Optional)
If you want to store file information in the database (e.g., filename, path, etc.), create a model and migration:
Bash
php artisan make:model File -m
Modify the File
model and migration to suit your needs.
3. Create a Form
Create a form in your Blade view with a file input field:
HTML
<form action="{{ route('file.upload') }}" method="POST" enctype="multipart/form-data">
@csrf
<input type="file" name="file">
<button type="submit">Upload</button>
</form>
Note the enctype="multipart/form-data"
attribute, essential for file uploads.
4. Define a Route
Create a route to handle the file upload:
PHP
Route::post('/upload', [UploadController::class, 'store'])->name('file.upload');
5. Create a Controller
Create a controller to handle the file upload logic:
PHP
use App\Http\Controllers\Controller;
use Illuminate\Http\Request;
use Illuminate\Support\Facades\Storage;
class UploadController extends Controller
{
public function store(Request $request)
{
// Validate the file
$request->validate([
'file' => 'required|file|mimes:jpeg,png,jpg,gif,pdf|max:2048' // Adjust validation rules as needed
]);
// Get the uploaded file
$file = $request->file('file');
// Generate a unique filename
$filename = time() . '_' . $file->getClientOriginalName();
// Store the file
$path = $file->storeAs('public/uploads', $filename);
// Optional: Save file information to database (if using a model)
// File::create(['path' => $path, ...]);
return redirect()->back()->with('success', 'File uploaded successfully');
}
}
6. Handle the Uploaded File
In the controller's store
method:
- Validate the file using Laravel's validation rules.
- Retrieve the uploaded file using
$request->file('file')
. - Generate a unique filename to avoid overwriting.
- Store the file using
Storage::putFileAs()
. - Optionally, save file information to the database if using a model.
- Return a success message or redirect to a desired page.
Additional Considerations
- File Validation: Implement robust validation to ensure file type, size, and other constraints.
- Error Handling: Handle potential errors during file upload and provide informative feedback to the user.
- Image Manipulation: Use libraries like Intervention Image to resize, crop, or manipulate images before storing.
- File Storage: Explore different storage options like Amazon S3 or other cloud storage providers.
- Security: Protect your application from vulnerabilities by sanitizing and validating user input.
Conclusion
By following these steps, you'll have a solid foundation for handling file uploads in your Laravel applications. Remember to adapt the code to your specific requirements and consider additional features like image optimization, file organization, and user permissions.